how to iterate through functions with button press in Swift
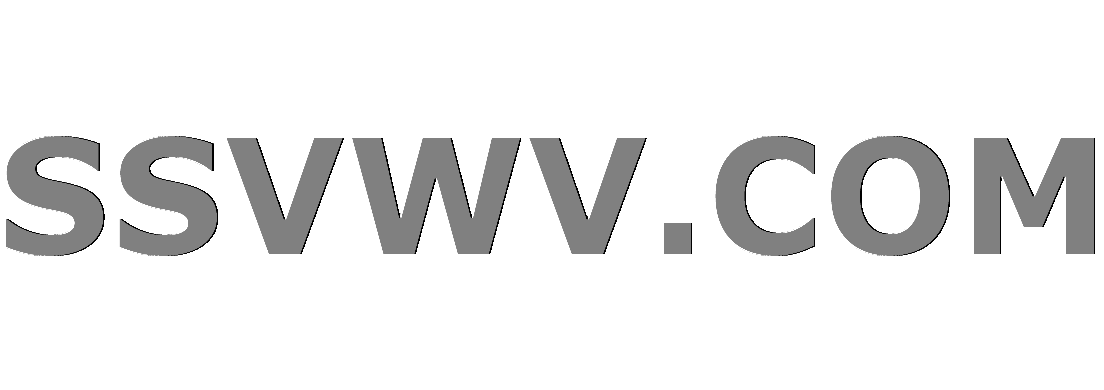
Multi tool use
I'm learning swift and building an ARApp but can't seem to understand how to iterate through a few functions with every separate button press. I have created 3 func with animation built in and want to press the button once and funcAnimation#1 activate then tap button again to proceed to funcAnimation#2 and so forth.
@IBAction func nextAnimation(_ sender: UIButton) {
funcAnimation#1()
funcAnimation#2()
funcAnimation#3()
}
of course the problem here is that they all activate at once. I would like to iterate only on button press for each individual press. In addition I would also like to have a backButton that reverses the current animation to previous animation. I read in Apple's documentation that there is a addTarget method but I don't understand how it works or how to implement it. Please Help!
ios swift
New contributor
Sam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
I'm learning swift and building an ARApp but can't seem to understand how to iterate through a few functions with every separate button press. I have created 3 func with animation built in and want to press the button once and funcAnimation#1 activate then tap button again to proceed to funcAnimation#2 and so forth.
@IBAction func nextAnimation(_ sender: UIButton) {
funcAnimation#1()
funcAnimation#2()
funcAnimation#3()
}
of course the problem here is that they all activate at once. I would like to iterate only on button press for each individual press. In addition I would also like to have a backButton that reverses the current animation to previous animation. I read in Apple's documentation that there is a addTarget method but I don't understand how it works or how to implement it. Please Help!
ios swift
New contributor
Sam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Use one counter (integer) argument which increment every time on button tap. Add one condition to set that counter to zero when it reaches to limit (ie. 3)
– Bhavik Modi
3 hours ago
You could also use completion handlers. Once a function finishes running, it runs the next.
– cyril
1 hour ago
add a comment |
I'm learning swift and building an ARApp but can't seem to understand how to iterate through a few functions with every separate button press. I have created 3 func with animation built in and want to press the button once and funcAnimation#1 activate then tap button again to proceed to funcAnimation#2 and so forth.
@IBAction func nextAnimation(_ sender: UIButton) {
funcAnimation#1()
funcAnimation#2()
funcAnimation#3()
}
of course the problem here is that they all activate at once. I would like to iterate only on button press for each individual press. In addition I would also like to have a backButton that reverses the current animation to previous animation. I read in Apple's documentation that there is a addTarget method but I don't understand how it works or how to implement it. Please Help!
ios swift
New contributor
Sam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I'm learning swift and building an ARApp but can't seem to understand how to iterate through a few functions with every separate button press. I have created 3 func with animation built in and want to press the button once and funcAnimation#1 activate then tap button again to proceed to funcAnimation#2 and so forth.
@IBAction func nextAnimation(_ sender: UIButton) {
funcAnimation#1()
funcAnimation#2()
funcAnimation#3()
}
of course the problem here is that they all activate at once. I would like to iterate only on button press for each individual press. In addition I would also like to have a backButton that reverses the current animation to previous animation. I read in Apple's documentation that there is a addTarget method but I don't understand how it works or how to implement it. Please Help!
ios swift
ios swift
New contributor
Sam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Sam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Sam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 3 hours ago
SamSam
332
332
New contributor
Sam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Sam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Sam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Use one counter (integer) argument which increment every time on button tap. Add one condition to set that counter to zero when it reaches to limit (ie. 3)
– Bhavik Modi
3 hours ago
You could also use completion handlers. Once a function finishes running, it runs the next.
– cyril
1 hour ago
add a comment |
Use one counter (integer) argument which increment every time on button tap. Add one condition to set that counter to zero when it reaches to limit (ie. 3)
– Bhavik Modi
3 hours ago
You could also use completion handlers. Once a function finishes running, it runs the next.
– cyril
1 hour ago
Use one counter (integer) argument which increment every time on button tap. Add one condition to set that counter to zero when it reaches to limit (ie. 3)
– Bhavik Modi
3 hours ago
Use one counter (integer) argument which increment every time on button tap. Add one condition to set that counter to zero when it reaches to limit (ie. 3)
– Bhavik Modi
3 hours ago
You could also use completion handlers. Once a function finishes running, it runs the next.
– cyril
1 hour ago
You could also use completion handlers. Once a function finishes running, it runs the next.
– cyril
1 hour ago
add a comment |
3 Answers
3
active
oldest
votes
Your code should like this :
// You can define this variable globally...
var counter = 0
@IBAction func nextAnimation(_ sender: UIButton) {
if (counter == 0) {
funcAnimation1()
// Increase counter count by 1 and you can add this line to completion of animation.
// You can also disable your button interaction until your animation gets complete and that way you can handle your UI
count += 1
}
else if (counter == 1) {
funcAnimation2()
// Increase counter count by 1 and you can add this line to completion of animation.
count += 1
}
else if (counter == 2) {
funcAnimation3()
// set your counter to 0 again to loop through your animation.
counter = 0
}
}
Your Back Action should look like this:
@IBAction func backAnimation(_ sender: UIButton) {
if (counter == 0) {
funcAnimation1()
// set your counter to 2 again to loop through your animation.
count = 2
}
else if (counter == 1) {
funcAnimation2()
// decrease counter count by 1 and you can add this line to completion of animation.
// You can also disable your button interaction until your animation gets complete and that way you can handle your UI
count -= 1
}
else if (counter == 2) {
funcAnimation3()
// decrease counter count by 1 and you can add this line to completion of animation.
count -= 1
}
}
You can disable the button after tap to complete the first animation and the second, then third just like that. It's all on you how you want to handle you UI flow.
– Jarvis The Avenger
3 hours ago
Thank you Jarvis, this worked great. and the animations occur individually on every button press. I have another quick question. I have two separate buttons, one "next" which applies the animations and one "back". Is there a way to reverse the current state of the animation to the previous animation? so let's say that you're on animation #3 and on "back" button it goes automatically to previous animation state #2?
– Sam
2 hours ago
Yes, you can do that by checking on Back button. decrease your counter by 1 in it and play the animation. but there is one breaking condition don't decrease your counter if counter == 0. Reset it to 2.
– Jarvis The Avenger
2 hours ago
@Sam I have updated my answer for your back button action.
– Jarvis The Avenger
2 hours ago
the back action does register that I'm pressing the back button and it is in theory applying that change but it is not reversing the animation. Since the animation has already happened when the "next" button was pressed then you don't see anything but your answer is registering in the console. I'm using SCNTransaction for the animation but there doesn't seem to be a .reversed method. I will continue to research that but I think that is another topic. I don't want to abuse your time. Your answers did help and were spot on. Thank you!
– Sam
2 hours ago
|
show 1 more comment
Another way!
Just set tag of button 1 (Ex. btnObj.tag=1
)
And manage method(s) in action of the button like
My suggestion is also manage flag
for animation like if 1st animation is in process then 2nd will be waiting for finish and after finishing 1st animation, button will be clicked so your animation will not mismatch.
var isAnimated = false;
@IBAction func myAnimationMethod(_ sender: UIButton) {
if isAnimated {return} // Or display appropriate message by alert
if (sender.tag == 1) {
isAnimated=true
funcAnimation1(...Your Code... After finish to set isAnimated=false)
sender.tag = 2
}
else if (sender.tag == 2) {
isAnimated=true
funcAnimation2(...Your Code... After finish to set isAnimated=false)
sender.tag = 3
}
else if (sender.tag == 3) {
isAnimated=true
funcAnimation3(...Your Code... After finish to set isAnimated=false)
sender.tag = 1
}
}
add a comment |
You can forward your animations and go back to previous animation state through a back button like this .
Step 1 : Declare two integer type variables
var tapCount = 0 //For forwarding your animations from first to third
var currentAnimation = 0 // For reversing the animation from current animation
Step 2 : In your IBAction Function
@IBAction func nextAnimation(_ sender: Any)
{
if tapCount == 0
{
if currentAnimation == 1
{
Animation2()
}
else
{
Animation1()
}
tapCount += 1
}
else if tapCount == 1
{
if currentAnimation == 2
{
Animation3()
}
else
{
Animation2()
}
tapCount += 1
}
else if tapCount == 2
{
if currentAnimation == 3
{
Animation1()
}
else
{
Animation3()
}
tapCount = 0
}
}
Step 3 : In your functions
func Animation1() {
currentAnimation = 1
print("First Animation")
}
func Animation2() {
currentAnimation = 2
print("Second Animation")
}
func Animation3() {
currentAnimation = 3
print("third Animation")
}
Step 4: Lastly For Reversing the animation from current state
@IBAction func backAnimation(_ sender: Any)
{
if currentAnimation == 2
{
Animation1()
}
else if currentAnimation == 3
{
Animation2()
}
else
{
}
tapCount = 0
}
Hope it helps !!
For addTarget(_:action:for:) method , this can be done without connecting a IBAction and declaring it in viewWillAppear or viewDidLoad as required.It will Associates a target object and action method with the control.
For example :
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
nextButton.addTarget(self, action: #selector(self. nextAnimation), for: .touchUpInside) //Declared outlet as nextButton
backButton.addTarget(self, action: #selector(self. backAnimation), for: .touchUpInside) //Declared outlet as backButton and this adds a target
}
@objc func nextAnimation(sender: UIButton) {
// Do your stuff for next animation
}
@objc func backAnimation(sender: UIButton) {
// Do your stuff for previous animation
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sam is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54319919%2fhow-to-iterate-through-functions-with-button-press-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your code should like this :
// You can define this variable globally...
var counter = 0
@IBAction func nextAnimation(_ sender: UIButton) {
if (counter == 0) {
funcAnimation1()
// Increase counter count by 1 and you can add this line to completion of animation.
// You can also disable your button interaction until your animation gets complete and that way you can handle your UI
count += 1
}
else if (counter == 1) {
funcAnimation2()
// Increase counter count by 1 and you can add this line to completion of animation.
count += 1
}
else if (counter == 2) {
funcAnimation3()
// set your counter to 0 again to loop through your animation.
counter = 0
}
}
Your Back Action should look like this:
@IBAction func backAnimation(_ sender: UIButton) {
if (counter == 0) {
funcAnimation1()
// set your counter to 2 again to loop through your animation.
count = 2
}
else if (counter == 1) {
funcAnimation2()
// decrease counter count by 1 and you can add this line to completion of animation.
// You can also disable your button interaction until your animation gets complete and that way you can handle your UI
count -= 1
}
else if (counter == 2) {
funcAnimation3()
// decrease counter count by 1 and you can add this line to completion of animation.
count -= 1
}
}
You can disable the button after tap to complete the first animation and the second, then third just like that. It's all on you how you want to handle you UI flow.
– Jarvis The Avenger
3 hours ago
Thank you Jarvis, this worked great. and the animations occur individually on every button press. I have another quick question. I have two separate buttons, one "next" which applies the animations and one "back". Is there a way to reverse the current state of the animation to the previous animation? so let's say that you're on animation #3 and on "back" button it goes automatically to previous animation state #2?
– Sam
2 hours ago
Yes, you can do that by checking on Back button. decrease your counter by 1 in it and play the animation. but there is one breaking condition don't decrease your counter if counter == 0. Reset it to 2.
– Jarvis The Avenger
2 hours ago
@Sam I have updated my answer for your back button action.
– Jarvis The Avenger
2 hours ago
the back action does register that I'm pressing the back button and it is in theory applying that change but it is not reversing the animation. Since the animation has already happened when the "next" button was pressed then you don't see anything but your answer is registering in the console. I'm using SCNTransaction for the animation but there doesn't seem to be a .reversed method. I will continue to research that but I think that is another topic. I don't want to abuse your time. Your answers did help and were spot on. Thank you!
– Sam
2 hours ago
|
show 1 more comment
Your code should like this :
// You can define this variable globally...
var counter = 0
@IBAction func nextAnimation(_ sender: UIButton) {
if (counter == 0) {
funcAnimation1()
// Increase counter count by 1 and you can add this line to completion of animation.
// You can also disable your button interaction until your animation gets complete and that way you can handle your UI
count += 1
}
else if (counter == 1) {
funcAnimation2()
// Increase counter count by 1 and you can add this line to completion of animation.
count += 1
}
else if (counter == 2) {
funcAnimation3()
// set your counter to 0 again to loop through your animation.
counter = 0
}
}
Your Back Action should look like this:
@IBAction func backAnimation(_ sender: UIButton) {
if (counter == 0) {
funcAnimation1()
// set your counter to 2 again to loop through your animation.
count = 2
}
else if (counter == 1) {
funcAnimation2()
// decrease counter count by 1 and you can add this line to completion of animation.
// You can also disable your button interaction until your animation gets complete and that way you can handle your UI
count -= 1
}
else if (counter == 2) {
funcAnimation3()
// decrease counter count by 1 and you can add this line to completion of animation.
count -= 1
}
}
You can disable the button after tap to complete the first animation and the second, then third just like that. It's all on you how you want to handle you UI flow.
– Jarvis The Avenger
3 hours ago
Thank you Jarvis, this worked great. and the animations occur individually on every button press. I have another quick question. I have two separate buttons, one "next" which applies the animations and one "back". Is there a way to reverse the current state of the animation to the previous animation? so let's say that you're on animation #3 and on "back" button it goes automatically to previous animation state #2?
– Sam
2 hours ago
Yes, you can do that by checking on Back button. decrease your counter by 1 in it and play the animation. but there is one breaking condition don't decrease your counter if counter == 0. Reset it to 2.
– Jarvis The Avenger
2 hours ago
@Sam I have updated my answer for your back button action.
– Jarvis The Avenger
2 hours ago
the back action does register that I'm pressing the back button and it is in theory applying that change but it is not reversing the animation. Since the animation has already happened when the "next" button was pressed then you don't see anything but your answer is registering in the console. I'm using SCNTransaction for the animation but there doesn't seem to be a .reversed method. I will continue to research that but I think that is another topic. I don't want to abuse your time. Your answers did help and were spot on. Thank you!
– Sam
2 hours ago
|
show 1 more comment
Your code should like this :
// You can define this variable globally...
var counter = 0
@IBAction func nextAnimation(_ sender: UIButton) {
if (counter == 0) {
funcAnimation1()
// Increase counter count by 1 and you can add this line to completion of animation.
// You can also disable your button interaction until your animation gets complete and that way you can handle your UI
count += 1
}
else if (counter == 1) {
funcAnimation2()
// Increase counter count by 1 and you can add this line to completion of animation.
count += 1
}
else if (counter == 2) {
funcAnimation3()
// set your counter to 0 again to loop through your animation.
counter = 0
}
}
Your Back Action should look like this:
@IBAction func backAnimation(_ sender: UIButton) {
if (counter == 0) {
funcAnimation1()
// set your counter to 2 again to loop through your animation.
count = 2
}
else if (counter == 1) {
funcAnimation2()
// decrease counter count by 1 and you can add this line to completion of animation.
// You can also disable your button interaction until your animation gets complete and that way you can handle your UI
count -= 1
}
else if (counter == 2) {
funcAnimation3()
// decrease counter count by 1 and you can add this line to completion of animation.
count -= 1
}
}
Your code should like this :
// You can define this variable globally...
var counter = 0
@IBAction func nextAnimation(_ sender: UIButton) {
if (counter == 0) {
funcAnimation1()
// Increase counter count by 1 and you can add this line to completion of animation.
// You can also disable your button interaction until your animation gets complete and that way you can handle your UI
count += 1
}
else if (counter == 1) {
funcAnimation2()
// Increase counter count by 1 and you can add this line to completion of animation.
count += 1
}
else if (counter == 2) {
funcAnimation3()
// set your counter to 0 again to loop through your animation.
counter = 0
}
}
Your Back Action should look like this:
@IBAction func backAnimation(_ sender: UIButton) {
if (counter == 0) {
funcAnimation1()
// set your counter to 2 again to loop through your animation.
count = 2
}
else if (counter == 1) {
funcAnimation2()
// decrease counter count by 1 and you can add this line to completion of animation.
// You can also disable your button interaction until your animation gets complete and that way you can handle your UI
count -= 1
}
else if (counter == 2) {
funcAnimation3()
// decrease counter count by 1 and you can add this line to completion of animation.
count -= 1
}
}
edited 2 hours ago
answered 3 hours ago
Jarvis The AvengerJarvis The Avenger
1,122916
1,122916
You can disable the button after tap to complete the first animation and the second, then third just like that. It's all on you how you want to handle you UI flow.
– Jarvis The Avenger
3 hours ago
Thank you Jarvis, this worked great. and the animations occur individually on every button press. I have another quick question. I have two separate buttons, one "next" which applies the animations and one "back". Is there a way to reverse the current state of the animation to the previous animation? so let's say that you're on animation #3 and on "back" button it goes automatically to previous animation state #2?
– Sam
2 hours ago
Yes, you can do that by checking on Back button. decrease your counter by 1 in it and play the animation. but there is one breaking condition don't decrease your counter if counter == 0. Reset it to 2.
– Jarvis The Avenger
2 hours ago
@Sam I have updated my answer for your back button action.
– Jarvis The Avenger
2 hours ago
the back action does register that I'm pressing the back button and it is in theory applying that change but it is not reversing the animation. Since the animation has already happened when the "next" button was pressed then you don't see anything but your answer is registering in the console. I'm using SCNTransaction for the animation but there doesn't seem to be a .reversed method. I will continue to research that but I think that is another topic. I don't want to abuse your time. Your answers did help and were spot on. Thank you!
– Sam
2 hours ago
|
show 1 more comment
You can disable the button after tap to complete the first animation and the second, then third just like that. It's all on you how you want to handle you UI flow.
– Jarvis The Avenger
3 hours ago
Thank you Jarvis, this worked great. and the animations occur individually on every button press. I have another quick question. I have two separate buttons, one "next" which applies the animations and one "back". Is there a way to reverse the current state of the animation to the previous animation? so let's say that you're on animation #3 and on "back" button it goes automatically to previous animation state #2?
– Sam
2 hours ago
Yes, you can do that by checking on Back button. decrease your counter by 1 in it and play the animation. but there is one breaking condition don't decrease your counter if counter == 0. Reset it to 2.
– Jarvis The Avenger
2 hours ago
@Sam I have updated my answer for your back button action.
– Jarvis The Avenger
2 hours ago
the back action does register that I'm pressing the back button and it is in theory applying that change but it is not reversing the animation. Since the animation has already happened when the "next" button was pressed then you don't see anything but your answer is registering in the console. I'm using SCNTransaction for the animation but there doesn't seem to be a .reversed method. I will continue to research that but I think that is another topic. I don't want to abuse your time. Your answers did help and were spot on. Thank you!
– Sam
2 hours ago
You can disable the button after tap to complete the first animation and the second, then third just like that. It's all on you how you want to handle you UI flow.
– Jarvis The Avenger
3 hours ago
You can disable the button after tap to complete the first animation and the second, then third just like that. It's all on you how you want to handle you UI flow.
– Jarvis The Avenger
3 hours ago
Thank you Jarvis, this worked great. and the animations occur individually on every button press. I have another quick question. I have two separate buttons, one "next" which applies the animations and one "back". Is there a way to reverse the current state of the animation to the previous animation? so let's say that you're on animation #3 and on "back" button it goes automatically to previous animation state #2?
– Sam
2 hours ago
Thank you Jarvis, this worked great. and the animations occur individually on every button press. I have another quick question. I have two separate buttons, one "next" which applies the animations and one "back". Is there a way to reverse the current state of the animation to the previous animation? so let's say that you're on animation #3 and on "back" button it goes automatically to previous animation state #2?
– Sam
2 hours ago
Yes, you can do that by checking on Back button. decrease your counter by 1 in it and play the animation. but there is one breaking condition don't decrease your counter if counter == 0. Reset it to 2.
– Jarvis The Avenger
2 hours ago
Yes, you can do that by checking on Back button. decrease your counter by 1 in it and play the animation. but there is one breaking condition don't decrease your counter if counter == 0. Reset it to 2.
– Jarvis The Avenger
2 hours ago
@Sam I have updated my answer for your back button action.
– Jarvis The Avenger
2 hours ago
@Sam I have updated my answer for your back button action.
– Jarvis The Avenger
2 hours ago
the back action does register that I'm pressing the back button and it is in theory applying that change but it is not reversing the animation. Since the animation has already happened when the "next" button was pressed then you don't see anything but your answer is registering in the console. I'm using SCNTransaction for the animation but there doesn't seem to be a .reversed method. I will continue to research that but I think that is another topic. I don't want to abuse your time. Your answers did help and were spot on. Thank you!
– Sam
2 hours ago
the back action does register that I'm pressing the back button and it is in theory applying that change but it is not reversing the animation. Since the animation has already happened when the "next" button was pressed then you don't see anything but your answer is registering in the console. I'm using SCNTransaction for the animation but there doesn't seem to be a .reversed method. I will continue to research that but I think that is another topic. I don't want to abuse your time. Your answers did help and were spot on. Thank you!
– Sam
2 hours ago
|
show 1 more comment
Another way!
Just set tag of button 1 (Ex. btnObj.tag=1
)
And manage method(s) in action of the button like
My suggestion is also manage flag
for animation like if 1st animation is in process then 2nd will be waiting for finish and after finishing 1st animation, button will be clicked so your animation will not mismatch.
var isAnimated = false;
@IBAction func myAnimationMethod(_ sender: UIButton) {
if isAnimated {return} // Or display appropriate message by alert
if (sender.tag == 1) {
isAnimated=true
funcAnimation1(...Your Code... After finish to set isAnimated=false)
sender.tag = 2
}
else if (sender.tag == 2) {
isAnimated=true
funcAnimation2(...Your Code... After finish to set isAnimated=false)
sender.tag = 3
}
else if (sender.tag == 3) {
isAnimated=true
funcAnimation3(...Your Code... After finish to set isAnimated=false)
sender.tag = 1
}
}
add a comment |
Another way!
Just set tag of button 1 (Ex. btnObj.tag=1
)
And manage method(s) in action of the button like
My suggestion is also manage flag
for animation like if 1st animation is in process then 2nd will be waiting for finish and after finishing 1st animation, button will be clicked so your animation will not mismatch.
var isAnimated = false;
@IBAction func myAnimationMethod(_ sender: UIButton) {
if isAnimated {return} // Or display appropriate message by alert
if (sender.tag == 1) {
isAnimated=true
funcAnimation1(...Your Code... After finish to set isAnimated=false)
sender.tag = 2
}
else if (sender.tag == 2) {
isAnimated=true
funcAnimation2(...Your Code... After finish to set isAnimated=false)
sender.tag = 3
}
else if (sender.tag == 3) {
isAnimated=true
funcAnimation3(...Your Code... After finish to set isAnimated=false)
sender.tag = 1
}
}
add a comment |
Another way!
Just set tag of button 1 (Ex. btnObj.tag=1
)
And manage method(s) in action of the button like
My suggestion is also manage flag
for animation like if 1st animation is in process then 2nd will be waiting for finish and after finishing 1st animation, button will be clicked so your animation will not mismatch.
var isAnimated = false;
@IBAction func myAnimationMethod(_ sender: UIButton) {
if isAnimated {return} // Or display appropriate message by alert
if (sender.tag == 1) {
isAnimated=true
funcAnimation1(...Your Code... After finish to set isAnimated=false)
sender.tag = 2
}
else if (sender.tag == 2) {
isAnimated=true
funcAnimation2(...Your Code... After finish to set isAnimated=false)
sender.tag = 3
}
else if (sender.tag == 3) {
isAnimated=true
funcAnimation3(...Your Code... After finish to set isAnimated=false)
sender.tag = 1
}
}
Another way!
Just set tag of button 1 (Ex. btnObj.tag=1
)
And manage method(s) in action of the button like
My suggestion is also manage flag
for animation like if 1st animation is in process then 2nd will be waiting for finish and after finishing 1st animation, button will be clicked so your animation will not mismatch.
var isAnimated = false;
@IBAction func myAnimationMethod(_ sender: UIButton) {
if isAnimated {return} // Or display appropriate message by alert
if (sender.tag == 1) {
isAnimated=true
funcAnimation1(...Your Code... After finish to set isAnimated=false)
sender.tag = 2
}
else if (sender.tag == 2) {
isAnimated=true
funcAnimation2(...Your Code... After finish to set isAnimated=false)
sender.tag = 3
}
else if (sender.tag == 3) {
isAnimated=true
funcAnimation3(...Your Code... After finish to set isAnimated=false)
sender.tag = 1
}
}
edited 2 hours ago


rmaddy
240k27315379
240k27315379
answered 2 hours ago


GovaadiyoGovaadiyo
921321
921321
add a comment |
add a comment |
You can forward your animations and go back to previous animation state through a back button like this .
Step 1 : Declare two integer type variables
var tapCount = 0 //For forwarding your animations from first to third
var currentAnimation = 0 // For reversing the animation from current animation
Step 2 : In your IBAction Function
@IBAction func nextAnimation(_ sender: Any)
{
if tapCount == 0
{
if currentAnimation == 1
{
Animation2()
}
else
{
Animation1()
}
tapCount += 1
}
else if tapCount == 1
{
if currentAnimation == 2
{
Animation3()
}
else
{
Animation2()
}
tapCount += 1
}
else if tapCount == 2
{
if currentAnimation == 3
{
Animation1()
}
else
{
Animation3()
}
tapCount = 0
}
}
Step 3 : In your functions
func Animation1() {
currentAnimation = 1
print("First Animation")
}
func Animation2() {
currentAnimation = 2
print("Second Animation")
}
func Animation3() {
currentAnimation = 3
print("third Animation")
}
Step 4: Lastly For Reversing the animation from current state
@IBAction func backAnimation(_ sender: Any)
{
if currentAnimation == 2
{
Animation1()
}
else if currentAnimation == 3
{
Animation2()
}
else
{
}
tapCount = 0
}
Hope it helps !!
For addTarget(_:action:for:) method , this can be done without connecting a IBAction and declaring it in viewWillAppear or viewDidLoad as required.It will Associates a target object and action method with the control.
For example :
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
nextButton.addTarget(self, action: #selector(self. nextAnimation), for: .touchUpInside) //Declared outlet as nextButton
backButton.addTarget(self, action: #selector(self. backAnimation), for: .touchUpInside) //Declared outlet as backButton and this adds a target
}
@objc func nextAnimation(sender: UIButton) {
// Do your stuff for next animation
}
@objc func backAnimation(sender: UIButton) {
// Do your stuff for previous animation
}
add a comment |
You can forward your animations and go back to previous animation state through a back button like this .
Step 1 : Declare two integer type variables
var tapCount = 0 //For forwarding your animations from first to third
var currentAnimation = 0 // For reversing the animation from current animation
Step 2 : In your IBAction Function
@IBAction func nextAnimation(_ sender: Any)
{
if tapCount == 0
{
if currentAnimation == 1
{
Animation2()
}
else
{
Animation1()
}
tapCount += 1
}
else if tapCount == 1
{
if currentAnimation == 2
{
Animation3()
}
else
{
Animation2()
}
tapCount += 1
}
else if tapCount == 2
{
if currentAnimation == 3
{
Animation1()
}
else
{
Animation3()
}
tapCount = 0
}
}
Step 3 : In your functions
func Animation1() {
currentAnimation = 1
print("First Animation")
}
func Animation2() {
currentAnimation = 2
print("Second Animation")
}
func Animation3() {
currentAnimation = 3
print("third Animation")
}
Step 4: Lastly For Reversing the animation from current state
@IBAction func backAnimation(_ sender: Any)
{
if currentAnimation == 2
{
Animation1()
}
else if currentAnimation == 3
{
Animation2()
}
else
{
}
tapCount = 0
}
Hope it helps !!
For addTarget(_:action:for:) method , this can be done without connecting a IBAction and declaring it in viewWillAppear or viewDidLoad as required.It will Associates a target object and action method with the control.
For example :
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
nextButton.addTarget(self, action: #selector(self. nextAnimation), for: .touchUpInside) //Declared outlet as nextButton
backButton.addTarget(self, action: #selector(self. backAnimation), for: .touchUpInside) //Declared outlet as backButton and this adds a target
}
@objc func nextAnimation(sender: UIButton) {
// Do your stuff for next animation
}
@objc func backAnimation(sender: UIButton) {
// Do your stuff for previous animation
}
add a comment |
You can forward your animations and go back to previous animation state through a back button like this .
Step 1 : Declare two integer type variables
var tapCount = 0 //For forwarding your animations from first to third
var currentAnimation = 0 // For reversing the animation from current animation
Step 2 : In your IBAction Function
@IBAction func nextAnimation(_ sender: Any)
{
if tapCount == 0
{
if currentAnimation == 1
{
Animation2()
}
else
{
Animation1()
}
tapCount += 1
}
else if tapCount == 1
{
if currentAnimation == 2
{
Animation3()
}
else
{
Animation2()
}
tapCount += 1
}
else if tapCount == 2
{
if currentAnimation == 3
{
Animation1()
}
else
{
Animation3()
}
tapCount = 0
}
}
Step 3 : In your functions
func Animation1() {
currentAnimation = 1
print("First Animation")
}
func Animation2() {
currentAnimation = 2
print("Second Animation")
}
func Animation3() {
currentAnimation = 3
print("third Animation")
}
Step 4: Lastly For Reversing the animation from current state
@IBAction func backAnimation(_ sender: Any)
{
if currentAnimation == 2
{
Animation1()
}
else if currentAnimation == 3
{
Animation2()
}
else
{
}
tapCount = 0
}
Hope it helps !!
For addTarget(_:action:for:) method , this can be done without connecting a IBAction and declaring it in viewWillAppear or viewDidLoad as required.It will Associates a target object and action method with the control.
For example :
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
nextButton.addTarget(self, action: #selector(self. nextAnimation), for: .touchUpInside) //Declared outlet as nextButton
backButton.addTarget(self, action: #selector(self. backAnimation), for: .touchUpInside) //Declared outlet as backButton and this adds a target
}
@objc func nextAnimation(sender: UIButton) {
// Do your stuff for next animation
}
@objc func backAnimation(sender: UIButton) {
// Do your stuff for previous animation
}
You can forward your animations and go back to previous animation state through a back button like this .
Step 1 : Declare two integer type variables
var tapCount = 0 //For forwarding your animations from first to third
var currentAnimation = 0 // For reversing the animation from current animation
Step 2 : In your IBAction Function
@IBAction func nextAnimation(_ sender: Any)
{
if tapCount == 0
{
if currentAnimation == 1
{
Animation2()
}
else
{
Animation1()
}
tapCount += 1
}
else if tapCount == 1
{
if currentAnimation == 2
{
Animation3()
}
else
{
Animation2()
}
tapCount += 1
}
else if tapCount == 2
{
if currentAnimation == 3
{
Animation1()
}
else
{
Animation3()
}
tapCount = 0
}
}
Step 3 : In your functions
func Animation1() {
currentAnimation = 1
print("First Animation")
}
func Animation2() {
currentAnimation = 2
print("Second Animation")
}
func Animation3() {
currentAnimation = 3
print("third Animation")
}
Step 4: Lastly For Reversing the animation from current state
@IBAction func backAnimation(_ sender: Any)
{
if currentAnimation == 2
{
Animation1()
}
else if currentAnimation == 3
{
Animation2()
}
else
{
}
tapCount = 0
}
Hope it helps !!
For addTarget(_:action:for:) method , this can be done without connecting a IBAction and declaring it in viewWillAppear or viewDidLoad as required.It will Associates a target object and action method with the control.
For example :
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
nextButton.addTarget(self, action: #selector(self. nextAnimation), for: .touchUpInside) //Declared outlet as nextButton
backButton.addTarget(self, action: #selector(self. backAnimation), for: .touchUpInside) //Declared outlet as backButton and this adds a target
}
@objc func nextAnimation(sender: UIButton) {
// Do your stuff for next animation
}
@objc func backAnimation(sender: UIButton) {
// Do your stuff for previous animation
}
edited 1 hour ago
answered 1 hour ago


Diksha BhargavaDiksha Bhargava
638
638
add a comment |
add a comment |
Sam is a new contributor. Be nice, and check out our Code of Conduct.
Sam is a new contributor. Be nice, and check out our Code of Conduct.
Sam is a new contributor. Be nice, and check out our Code of Conduct.
Sam is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54319919%2fhow-to-iterate-through-functions-with-button-press-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
P6,TvL8pmBhPDH0pd9sRoDbH4JNDpkVOOs7BI6R6ICMWhg4zu0F,zFZa0t6Y7zwl,e
Use one counter (integer) argument which increment every time on button tap. Add one condition to set that counter to zero when it reaches to limit (ie. 3)
– Bhavik Modi
3 hours ago
You could also use completion handlers. Once a function finishes running, it runs the next.
– cyril
1 hour ago