Replicate or Call java encryption function in Oracle pl/sql
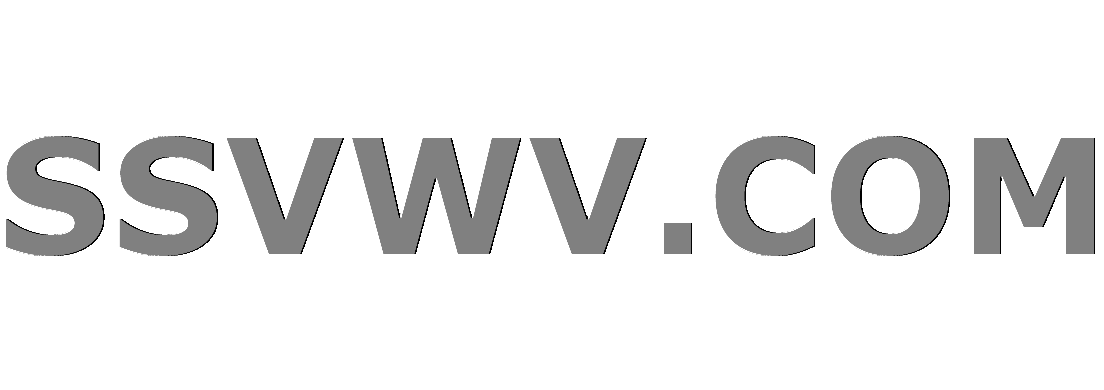
Multi tool use
I am trying to replicate encryption/decryption method present in java in Oracle DB, so that data encrypted in java can be decrypted through Oracle Function.
Below is java code:
package com.encr;
import java.security.NoSuchAlgorithmException;
import javax.crypto.Cipher;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import sun.misc.BASE64Decoder;
import sun.misc.BASE64Encoder;
public class EncrUtil
{
private static Cipher cipher = null;
public static void aesInit(String inKey) throws Exception
{
String methodName = "aesInit";
try
{
byte inkeyBytes = inKey.getBytes("utf-8");
System.out.println("inkeyBytes="+inkeyBytes.toString());
final SecretKeySpec clientkey = new SecretKeySpec(inkeyBytes, "AES");
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
final IvParameterSpec iv = new IvParameterSpec(new byte[32]);
cipher.init(Cipher.ENCRYPT_MODE,clientkey,iv);
}
catch (NoSuchAlgorithmException e) {
throw new Exception("NoSuchAlgorithmException", e);
} catch (NoSuchPaddingException e) {
throw new Exception("NoSuchPaddingException", e);
}
}
public static String encrypt(String inData,String inKey)
{
String methodName = "encrypt";
String strCipherText = null;
try
{
if ( null != inData && null != inKey)
{
if(EncrUtil.cipher == null){
EncrUtil.aesInit(inKey);
}
byte byteDataToEncrypt = inData.getBytes("utf-8");
System.out.println(byteDataToEncrypt.toString());
byte byteCipherText = cipher.doFinal(byteDataToEncrypt);
System.out.println(byteCipherText.toString());
strCipherText = new BASE64Encoder().encode(byteCipherText);
}
}
catch (Exception e) {
String sErrMsg = "Text to be encrypted: " + inData;
sErrMsg = new StringBuffer(methodName).append( sErrMsg) .append( " Message: ") .append(e.getMessage()).toString();
strCipherText = sErrMsg;
}
return strCipherText;
}
public static String decrypt(String inData,String inKey)
{
String methodName = "decrypt";
String strDecryptedText = null;
try
{
byte inkeyBytes = inKey.getBytes("utf-8");
final SecretKeySpec clientkey = new SecretKeySpec(inkeyBytes, "AES");
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
final IvParameterSpec iv = new IvParameterSpec(new byte[16]);
cipher.init(Cipher.DECRYPT_MODE,clientkey,iv);
byte decodedData = new BASE64Decoder().decodeBuffer(inData);
byte byteDecryptedText = cipher.doFinal(decodedData);
strDecryptedText = new String(byteDecryptedText);
}
catch (Exception e) {
String sErrMsg = "Text to be decrypted: " + inData;
sErrMsg = new StringBuffer(methodName).append(sErrMsg) .append( "Message:") .append(e.getMessage()).toString();
strDecryptedText = sErrMsg;
}
return strDecryptedText;
}
public static void main(String args)
{
String inKey= "84hf763bht096hnf";
String inData= "Vikram";
System.out.println("Word to be encrypted is "+inData);
String encrypted = EncrUtil.encrypt(inData,inKey);
System.out.println("Encrpted word is"+encrypted);
}
}
The Oracle Pl/sql function i tried for replicating is
CREATE OR REPLACE PACKAGE BODY SYS.enc_dec
AS
encryption_type PLS_INTEGER := DBMS_CRYPTO.ENCRYPT_AES
+ DBMS_CRYPTO.CHAIN_CBC
+ DBMS_CRYPTO.PAD_PKCS5;
encryption_key RAW (32) := UTL_I18N.STRING_TO_RAW ('84hf763bht096hnf', 'AL32UTF8');
FUNCTION encrypt (p_plainText VARCHAR2) RETURN RAW DETERMINISTIC
IS
encrypted_raw RAW (2000);
BEGIN
DBMS_OUTPUT.PUT_LINE(UTL_RAW.CAST_TO_VARCHAR2(encryption_key));
encrypted_raw := DBMS_CRYPTO.ENCRYPT
(
--src => UTL_RAW.CAST_TO_RAW (p_plainText),
src =>UTL_I18N.STRING_TO_RAW (p_plainText,'AL32UTF8'),
typ => encryption_type,
key => encryption_key
);
RETURN encrypted_raw;
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE(SUBSTR(SQLERRM, 1, 100));
END encrypt;
FUNCTION decrypt (p_encryptedText RAW) RETURN VARCHAR2 DETERMINISTIC
IS
decrypted_raw RAW (2000);
BEGIN
decrypted_raw := DBMS_CRYPTO.DECRYPT
(
src => p_encryptedText,
typ => encryption_type,
key => encryption_key
);
RETURN (UTL_I18N.RAW_TO_CHAR(decrypted_raw,'AL16UTF8'));
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE(SUBSTR(SQLERRM, 1, 100));
END decrypt;
END;
Encryption done by above code is not same as that done in java
Please let me know where i am going wrong in oracle function or
Please tell me how to add the java methos in oracle, in tutorials i couldn't find how to include imports of java in oracle.
oracle-11g plsql encryption java
add a comment |
I am trying to replicate encryption/decryption method present in java in Oracle DB, so that data encrypted in java can be decrypted through Oracle Function.
Below is java code:
package com.encr;
import java.security.NoSuchAlgorithmException;
import javax.crypto.Cipher;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import sun.misc.BASE64Decoder;
import sun.misc.BASE64Encoder;
public class EncrUtil
{
private static Cipher cipher = null;
public static void aesInit(String inKey) throws Exception
{
String methodName = "aesInit";
try
{
byte inkeyBytes = inKey.getBytes("utf-8");
System.out.println("inkeyBytes="+inkeyBytes.toString());
final SecretKeySpec clientkey = new SecretKeySpec(inkeyBytes, "AES");
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
final IvParameterSpec iv = new IvParameterSpec(new byte[32]);
cipher.init(Cipher.ENCRYPT_MODE,clientkey,iv);
}
catch (NoSuchAlgorithmException e) {
throw new Exception("NoSuchAlgorithmException", e);
} catch (NoSuchPaddingException e) {
throw new Exception("NoSuchPaddingException", e);
}
}
public static String encrypt(String inData,String inKey)
{
String methodName = "encrypt";
String strCipherText = null;
try
{
if ( null != inData && null != inKey)
{
if(EncrUtil.cipher == null){
EncrUtil.aesInit(inKey);
}
byte byteDataToEncrypt = inData.getBytes("utf-8");
System.out.println(byteDataToEncrypt.toString());
byte byteCipherText = cipher.doFinal(byteDataToEncrypt);
System.out.println(byteCipherText.toString());
strCipherText = new BASE64Encoder().encode(byteCipherText);
}
}
catch (Exception e) {
String sErrMsg = "Text to be encrypted: " + inData;
sErrMsg = new StringBuffer(methodName).append( sErrMsg) .append( " Message: ") .append(e.getMessage()).toString();
strCipherText = sErrMsg;
}
return strCipherText;
}
public static String decrypt(String inData,String inKey)
{
String methodName = "decrypt";
String strDecryptedText = null;
try
{
byte inkeyBytes = inKey.getBytes("utf-8");
final SecretKeySpec clientkey = new SecretKeySpec(inkeyBytes, "AES");
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
final IvParameterSpec iv = new IvParameterSpec(new byte[16]);
cipher.init(Cipher.DECRYPT_MODE,clientkey,iv);
byte decodedData = new BASE64Decoder().decodeBuffer(inData);
byte byteDecryptedText = cipher.doFinal(decodedData);
strDecryptedText = new String(byteDecryptedText);
}
catch (Exception e) {
String sErrMsg = "Text to be decrypted: " + inData;
sErrMsg = new StringBuffer(methodName).append(sErrMsg) .append( "Message:") .append(e.getMessage()).toString();
strDecryptedText = sErrMsg;
}
return strDecryptedText;
}
public static void main(String args)
{
String inKey= "84hf763bht096hnf";
String inData= "Vikram";
System.out.println("Word to be encrypted is "+inData);
String encrypted = EncrUtil.encrypt(inData,inKey);
System.out.println("Encrpted word is"+encrypted);
}
}
The Oracle Pl/sql function i tried for replicating is
CREATE OR REPLACE PACKAGE BODY SYS.enc_dec
AS
encryption_type PLS_INTEGER := DBMS_CRYPTO.ENCRYPT_AES
+ DBMS_CRYPTO.CHAIN_CBC
+ DBMS_CRYPTO.PAD_PKCS5;
encryption_key RAW (32) := UTL_I18N.STRING_TO_RAW ('84hf763bht096hnf', 'AL32UTF8');
FUNCTION encrypt (p_plainText VARCHAR2) RETURN RAW DETERMINISTIC
IS
encrypted_raw RAW (2000);
BEGIN
DBMS_OUTPUT.PUT_LINE(UTL_RAW.CAST_TO_VARCHAR2(encryption_key));
encrypted_raw := DBMS_CRYPTO.ENCRYPT
(
--src => UTL_RAW.CAST_TO_RAW (p_plainText),
src =>UTL_I18N.STRING_TO_RAW (p_plainText,'AL32UTF8'),
typ => encryption_type,
key => encryption_key
);
RETURN encrypted_raw;
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE(SUBSTR(SQLERRM, 1, 100));
END encrypt;
FUNCTION decrypt (p_encryptedText RAW) RETURN VARCHAR2 DETERMINISTIC
IS
decrypted_raw RAW (2000);
BEGIN
decrypted_raw := DBMS_CRYPTO.DECRYPT
(
src => p_encryptedText,
typ => encryption_type,
key => encryption_key
);
RETURN (UTL_I18N.RAW_TO_CHAR(decrypted_raw,'AL16UTF8'));
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE(SUBSTR(SQLERRM, 1, 100));
END decrypt;
END;
Encryption done by above code is not same as that done in java
Please let me know where i am going wrong in oracle function or
Please tell me how to add the java methos in oracle, in tutorials i couldn't find how to include imports of java in oracle.
oracle-11g plsql encryption java
add a comment |
I am trying to replicate encryption/decryption method present in java in Oracle DB, so that data encrypted in java can be decrypted through Oracle Function.
Below is java code:
package com.encr;
import java.security.NoSuchAlgorithmException;
import javax.crypto.Cipher;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import sun.misc.BASE64Decoder;
import sun.misc.BASE64Encoder;
public class EncrUtil
{
private static Cipher cipher = null;
public static void aesInit(String inKey) throws Exception
{
String methodName = "aesInit";
try
{
byte inkeyBytes = inKey.getBytes("utf-8");
System.out.println("inkeyBytes="+inkeyBytes.toString());
final SecretKeySpec clientkey = new SecretKeySpec(inkeyBytes, "AES");
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
final IvParameterSpec iv = new IvParameterSpec(new byte[32]);
cipher.init(Cipher.ENCRYPT_MODE,clientkey,iv);
}
catch (NoSuchAlgorithmException e) {
throw new Exception("NoSuchAlgorithmException", e);
} catch (NoSuchPaddingException e) {
throw new Exception("NoSuchPaddingException", e);
}
}
public static String encrypt(String inData,String inKey)
{
String methodName = "encrypt";
String strCipherText = null;
try
{
if ( null != inData && null != inKey)
{
if(EncrUtil.cipher == null){
EncrUtil.aesInit(inKey);
}
byte byteDataToEncrypt = inData.getBytes("utf-8");
System.out.println(byteDataToEncrypt.toString());
byte byteCipherText = cipher.doFinal(byteDataToEncrypt);
System.out.println(byteCipherText.toString());
strCipherText = new BASE64Encoder().encode(byteCipherText);
}
}
catch (Exception e) {
String sErrMsg = "Text to be encrypted: " + inData;
sErrMsg = new StringBuffer(methodName).append( sErrMsg) .append( " Message: ") .append(e.getMessage()).toString();
strCipherText = sErrMsg;
}
return strCipherText;
}
public static String decrypt(String inData,String inKey)
{
String methodName = "decrypt";
String strDecryptedText = null;
try
{
byte inkeyBytes = inKey.getBytes("utf-8");
final SecretKeySpec clientkey = new SecretKeySpec(inkeyBytes, "AES");
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
final IvParameterSpec iv = new IvParameterSpec(new byte[16]);
cipher.init(Cipher.DECRYPT_MODE,clientkey,iv);
byte decodedData = new BASE64Decoder().decodeBuffer(inData);
byte byteDecryptedText = cipher.doFinal(decodedData);
strDecryptedText = new String(byteDecryptedText);
}
catch (Exception e) {
String sErrMsg = "Text to be decrypted: " + inData;
sErrMsg = new StringBuffer(methodName).append(sErrMsg) .append( "Message:") .append(e.getMessage()).toString();
strDecryptedText = sErrMsg;
}
return strDecryptedText;
}
public static void main(String args)
{
String inKey= "84hf763bht096hnf";
String inData= "Vikram";
System.out.println("Word to be encrypted is "+inData);
String encrypted = EncrUtil.encrypt(inData,inKey);
System.out.println("Encrpted word is"+encrypted);
}
}
The Oracle Pl/sql function i tried for replicating is
CREATE OR REPLACE PACKAGE BODY SYS.enc_dec
AS
encryption_type PLS_INTEGER := DBMS_CRYPTO.ENCRYPT_AES
+ DBMS_CRYPTO.CHAIN_CBC
+ DBMS_CRYPTO.PAD_PKCS5;
encryption_key RAW (32) := UTL_I18N.STRING_TO_RAW ('84hf763bht096hnf', 'AL32UTF8');
FUNCTION encrypt (p_plainText VARCHAR2) RETURN RAW DETERMINISTIC
IS
encrypted_raw RAW (2000);
BEGIN
DBMS_OUTPUT.PUT_LINE(UTL_RAW.CAST_TO_VARCHAR2(encryption_key));
encrypted_raw := DBMS_CRYPTO.ENCRYPT
(
--src => UTL_RAW.CAST_TO_RAW (p_plainText),
src =>UTL_I18N.STRING_TO_RAW (p_plainText,'AL32UTF8'),
typ => encryption_type,
key => encryption_key
);
RETURN encrypted_raw;
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE(SUBSTR(SQLERRM, 1, 100));
END encrypt;
FUNCTION decrypt (p_encryptedText RAW) RETURN VARCHAR2 DETERMINISTIC
IS
decrypted_raw RAW (2000);
BEGIN
decrypted_raw := DBMS_CRYPTO.DECRYPT
(
src => p_encryptedText,
typ => encryption_type,
key => encryption_key
);
RETURN (UTL_I18N.RAW_TO_CHAR(decrypted_raw,'AL16UTF8'));
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE(SUBSTR(SQLERRM, 1, 100));
END decrypt;
END;
Encryption done by above code is not same as that done in java
Please let me know where i am going wrong in oracle function or
Please tell me how to add the java methos in oracle, in tutorials i couldn't find how to include imports of java in oracle.
oracle-11g plsql encryption java
I am trying to replicate encryption/decryption method present in java in Oracle DB, so that data encrypted in java can be decrypted through Oracle Function.
Below is java code:
package com.encr;
import java.security.NoSuchAlgorithmException;
import javax.crypto.Cipher;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import sun.misc.BASE64Decoder;
import sun.misc.BASE64Encoder;
public class EncrUtil
{
private static Cipher cipher = null;
public static void aesInit(String inKey) throws Exception
{
String methodName = "aesInit";
try
{
byte inkeyBytes = inKey.getBytes("utf-8");
System.out.println("inkeyBytes="+inkeyBytes.toString());
final SecretKeySpec clientkey = new SecretKeySpec(inkeyBytes, "AES");
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
final IvParameterSpec iv = new IvParameterSpec(new byte[32]);
cipher.init(Cipher.ENCRYPT_MODE,clientkey,iv);
}
catch (NoSuchAlgorithmException e) {
throw new Exception("NoSuchAlgorithmException", e);
} catch (NoSuchPaddingException e) {
throw new Exception("NoSuchPaddingException", e);
}
}
public static String encrypt(String inData,String inKey)
{
String methodName = "encrypt";
String strCipherText = null;
try
{
if ( null != inData && null != inKey)
{
if(EncrUtil.cipher == null){
EncrUtil.aesInit(inKey);
}
byte byteDataToEncrypt = inData.getBytes("utf-8");
System.out.println(byteDataToEncrypt.toString());
byte byteCipherText = cipher.doFinal(byteDataToEncrypt);
System.out.println(byteCipherText.toString());
strCipherText = new BASE64Encoder().encode(byteCipherText);
}
}
catch (Exception e) {
String sErrMsg = "Text to be encrypted: " + inData;
sErrMsg = new StringBuffer(methodName).append( sErrMsg) .append( " Message: ") .append(e.getMessage()).toString();
strCipherText = sErrMsg;
}
return strCipherText;
}
public static String decrypt(String inData,String inKey)
{
String methodName = "decrypt";
String strDecryptedText = null;
try
{
byte inkeyBytes = inKey.getBytes("utf-8");
final SecretKeySpec clientkey = new SecretKeySpec(inkeyBytes, "AES");
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
final IvParameterSpec iv = new IvParameterSpec(new byte[16]);
cipher.init(Cipher.DECRYPT_MODE,clientkey,iv);
byte decodedData = new BASE64Decoder().decodeBuffer(inData);
byte byteDecryptedText = cipher.doFinal(decodedData);
strDecryptedText = new String(byteDecryptedText);
}
catch (Exception e) {
String sErrMsg = "Text to be decrypted: " + inData;
sErrMsg = new StringBuffer(methodName).append(sErrMsg) .append( "Message:") .append(e.getMessage()).toString();
strDecryptedText = sErrMsg;
}
return strDecryptedText;
}
public static void main(String args)
{
String inKey= "84hf763bht096hnf";
String inData= "Vikram";
System.out.println("Word to be encrypted is "+inData);
String encrypted = EncrUtil.encrypt(inData,inKey);
System.out.println("Encrpted word is"+encrypted);
}
}
The Oracle Pl/sql function i tried for replicating is
CREATE OR REPLACE PACKAGE BODY SYS.enc_dec
AS
encryption_type PLS_INTEGER := DBMS_CRYPTO.ENCRYPT_AES
+ DBMS_CRYPTO.CHAIN_CBC
+ DBMS_CRYPTO.PAD_PKCS5;
encryption_key RAW (32) := UTL_I18N.STRING_TO_RAW ('84hf763bht096hnf', 'AL32UTF8');
FUNCTION encrypt (p_plainText VARCHAR2) RETURN RAW DETERMINISTIC
IS
encrypted_raw RAW (2000);
BEGIN
DBMS_OUTPUT.PUT_LINE(UTL_RAW.CAST_TO_VARCHAR2(encryption_key));
encrypted_raw := DBMS_CRYPTO.ENCRYPT
(
--src => UTL_RAW.CAST_TO_RAW (p_plainText),
src =>UTL_I18N.STRING_TO_RAW (p_plainText,'AL32UTF8'),
typ => encryption_type,
key => encryption_key
);
RETURN encrypted_raw;
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE(SUBSTR(SQLERRM, 1, 100));
END encrypt;
FUNCTION decrypt (p_encryptedText RAW) RETURN VARCHAR2 DETERMINISTIC
IS
decrypted_raw RAW (2000);
BEGIN
decrypted_raw := DBMS_CRYPTO.DECRYPT
(
src => p_encryptedText,
typ => encryption_type,
key => encryption_key
);
RETURN (UTL_I18N.RAW_TO_CHAR(decrypted_raw,'AL16UTF8'));
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE(SUBSTR(SQLERRM, 1, 100));
END decrypt;
END;
Encryption done by above code is not same as that done in java
Please let me know where i am going wrong in oracle function or
Please tell me how to add the java methos in oracle, in tutorials i couldn't find how to include imports of java in oracle.
oracle-11g plsql encryption java
oracle-11g plsql encryption java
edited Oct 9 '18 at 9:54
a_horse_with_no_name
39.1k775112
39.1k775112
asked Aug 7 '13 at 9:13
Vikram VVikram V
5614
5614
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
I was able to move the Java code to Oracle. I am writing this for people who are facing similar issue.
- Log on to server where Oracle database is installed through Putty (command prompt in case of windows)
- Place the Java file in server using WinSCP.
Find the Java compiler present in oracle home using command
find / -name javac
Compile the Java file using Oracle's Java compiler as the Java version used by the database and Java version usually used will be different
$ORACLE_HOME/jdk/bin/javac /home/vikram/EncrUtil.java
Note: Instead of compiling on the database server, a
.class
or.jar
file can be directly loaded into the database. But make sure to use the same version of Java as used by the database.
To find the java version used by oracle
$ORACLE_HOME/jdk/bin/java -version
java version "1.5.0_17"
Java(TM) 2 Runtime Environment, Standard Edition (build 1.5.0_17-b03)
Java HotSpot(TM) 64-Bit Server VM (build 1.5.0_17-b03, mixed mode)
Set ORACLE_HOME if it is not set
export ORACLE_HOME=/database/ora11gr2/product/11.2.0/dbhome_1/
Add
loadjava
's directory in the path (that's the database's bin directory)
export PATH=$PATH:$ORACLE_HOME/bin
Note: In case class not found exception is found go through “loadjava” and check if path present is properly set or variables are defined is not define those
Load the class into the database
loadjava -user <user>/<password>@xxx.xxx.xxx.xxxx:1521:<instance>
-thin –resolve /home/vikram/ EncrUtil.class
-resolve
is used so that.class
file can be resolved before loading into the database as its easy to debug Java outside database.
-thin
is used for thin client
Once the class is loaded, create a wrapper function on top it, so that it can be used in database
CREATE OR REPLACE FUNCTION TEST_DECRYPTOR(indata IN VARCHAR2)
RETURN VARCHAR2 AS
LANGUAGE JAVA NAME ' EncrUtil. decrypt (java.lang.String) return java.lang.String';
CREATE OR REPLACE FUNCTION TEST_ENCRYPTOR (indata IN VARCHAR2)
RETURN VARCHAR2 AS
LANGUAGE JAVA NAME ' EncrUtil. encrypt (java.lang.String) return java.lang.String';
This function can then be used just like any other Oracle function:
SELECT TEST_ENCRYPTOR('Vikram') FROM DUAL;
add a comment |
A little late but the answer may be useful:
In Java this line should be changed:
final IvParameterSpec iv = new IvParameterSpec(new byte[16]);
into this:
final IvParameterSpec iv = new IvParameterSpec(new String(Base64.decode('vector_inicializacion')).getBytes());
where vector_inicializacion is a any hexadecimal of 16 but in PL/SQL it must be the same.
and
strDecryptedText = new String(byteDecryptedText);
Should be replaced by
strDecryptedText = new String(Base64.encodeBytes(byteDecryptedText))
In PL:
encrypted_raw := DBMS_CRYPTO.ENCRYPT
(
--src => UTL_RAW.CAST_TO_RAW (p_plainText),
src =>UTL_I18N.STRING_TO_RAW (p_plainText,'AL32UTF8'),
typ => encryption_type,
key => encryption_key
IV => UTL_RAW.CAST_TO_RAW ('vector_inicializacion')
);
add a comment |
ORA-29531: no method encrypt in class EncrUtil
why this error may appear?
New contributor
Oktay Aliyev is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "182"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fdba.stackexchange.com%2fquestions%2f47696%2freplicate-or-call-java-encryption-function-in-oracle-pl-sql%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
I was able to move the Java code to Oracle. I am writing this for people who are facing similar issue.
- Log on to server where Oracle database is installed through Putty (command prompt in case of windows)
- Place the Java file in server using WinSCP.
Find the Java compiler present in oracle home using command
find / -name javac
Compile the Java file using Oracle's Java compiler as the Java version used by the database and Java version usually used will be different
$ORACLE_HOME/jdk/bin/javac /home/vikram/EncrUtil.java
Note: Instead of compiling on the database server, a
.class
or.jar
file can be directly loaded into the database. But make sure to use the same version of Java as used by the database.
To find the java version used by oracle
$ORACLE_HOME/jdk/bin/java -version
java version "1.5.0_17"
Java(TM) 2 Runtime Environment, Standard Edition (build 1.5.0_17-b03)
Java HotSpot(TM) 64-Bit Server VM (build 1.5.0_17-b03, mixed mode)
Set ORACLE_HOME if it is not set
export ORACLE_HOME=/database/ora11gr2/product/11.2.0/dbhome_1/
Add
loadjava
's directory in the path (that's the database's bin directory)
export PATH=$PATH:$ORACLE_HOME/bin
Note: In case class not found exception is found go through “loadjava” and check if path present is properly set or variables are defined is not define those
Load the class into the database
loadjava -user <user>/<password>@xxx.xxx.xxx.xxxx:1521:<instance>
-thin –resolve /home/vikram/ EncrUtil.class
-resolve
is used so that.class
file can be resolved before loading into the database as its easy to debug Java outside database.
-thin
is used for thin client
Once the class is loaded, create a wrapper function on top it, so that it can be used in database
CREATE OR REPLACE FUNCTION TEST_DECRYPTOR(indata IN VARCHAR2)
RETURN VARCHAR2 AS
LANGUAGE JAVA NAME ' EncrUtil. decrypt (java.lang.String) return java.lang.String';
CREATE OR REPLACE FUNCTION TEST_ENCRYPTOR (indata IN VARCHAR2)
RETURN VARCHAR2 AS
LANGUAGE JAVA NAME ' EncrUtil. encrypt (java.lang.String) return java.lang.String';
This function can then be used just like any other Oracle function:
SELECT TEST_ENCRYPTOR('Vikram') FROM DUAL;
add a comment |
I was able to move the Java code to Oracle. I am writing this for people who are facing similar issue.
- Log on to server where Oracle database is installed through Putty (command prompt in case of windows)
- Place the Java file in server using WinSCP.
Find the Java compiler present in oracle home using command
find / -name javac
Compile the Java file using Oracle's Java compiler as the Java version used by the database and Java version usually used will be different
$ORACLE_HOME/jdk/bin/javac /home/vikram/EncrUtil.java
Note: Instead of compiling on the database server, a
.class
or.jar
file can be directly loaded into the database. But make sure to use the same version of Java as used by the database.
To find the java version used by oracle
$ORACLE_HOME/jdk/bin/java -version
java version "1.5.0_17"
Java(TM) 2 Runtime Environment, Standard Edition (build 1.5.0_17-b03)
Java HotSpot(TM) 64-Bit Server VM (build 1.5.0_17-b03, mixed mode)
Set ORACLE_HOME if it is not set
export ORACLE_HOME=/database/ora11gr2/product/11.2.0/dbhome_1/
Add
loadjava
's directory in the path (that's the database's bin directory)
export PATH=$PATH:$ORACLE_HOME/bin
Note: In case class not found exception is found go through “loadjava” and check if path present is properly set or variables are defined is not define those
Load the class into the database
loadjava -user <user>/<password>@xxx.xxx.xxx.xxxx:1521:<instance>
-thin –resolve /home/vikram/ EncrUtil.class
-resolve
is used so that.class
file can be resolved before loading into the database as its easy to debug Java outside database.
-thin
is used for thin client
Once the class is loaded, create a wrapper function on top it, so that it can be used in database
CREATE OR REPLACE FUNCTION TEST_DECRYPTOR(indata IN VARCHAR2)
RETURN VARCHAR2 AS
LANGUAGE JAVA NAME ' EncrUtil. decrypt (java.lang.String) return java.lang.String';
CREATE OR REPLACE FUNCTION TEST_ENCRYPTOR (indata IN VARCHAR2)
RETURN VARCHAR2 AS
LANGUAGE JAVA NAME ' EncrUtil. encrypt (java.lang.String) return java.lang.String';
This function can then be used just like any other Oracle function:
SELECT TEST_ENCRYPTOR('Vikram') FROM DUAL;
add a comment |
I was able to move the Java code to Oracle. I am writing this for people who are facing similar issue.
- Log on to server where Oracle database is installed through Putty (command prompt in case of windows)
- Place the Java file in server using WinSCP.
Find the Java compiler present in oracle home using command
find / -name javac
Compile the Java file using Oracle's Java compiler as the Java version used by the database and Java version usually used will be different
$ORACLE_HOME/jdk/bin/javac /home/vikram/EncrUtil.java
Note: Instead of compiling on the database server, a
.class
or.jar
file can be directly loaded into the database. But make sure to use the same version of Java as used by the database.
To find the java version used by oracle
$ORACLE_HOME/jdk/bin/java -version
java version "1.5.0_17"
Java(TM) 2 Runtime Environment, Standard Edition (build 1.5.0_17-b03)
Java HotSpot(TM) 64-Bit Server VM (build 1.5.0_17-b03, mixed mode)
Set ORACLE_HOME if it is not set
export ORACLE_HOME=/database/ora11gr2/product/11.2.0/dbhome_1/
Add
loadjava
's directory in the path (that's the database's bin directory)
export PATH=$PATH:$ORACLE_HOME/bin
Note: In case class not found exception is found go through “loadjava” and check if path present is properly set or variables are defined is not define those
Load the class into the database
loadjava -user <user>/<password>@xxx.xxx.xxx.xxxx:1521:<instance>
-thin –resolve /home/vikram/ EncrUtil.class
-resolve
is used so that.class
file can be resolved before loading into the database as its easy to debug Java outside database.
-thin
is used for thin client
Once the class is loaded, create a wrapper function on top it, so that it can be used in database
CREATE OR REPLACE FUNCTION TEST_DECRYPTOR(indata IN VARCHAR2)
RETURN VARCHAR2 AS
LANGUAGE JAVA NAME ' EncrUtil. decrypt (java.lang.String) return java.lang.String';
CREATE OR REPLACE FUNCTION TEST_ENCRYPTOR (indata IN VARCHAR2)
RETURN VARCHAR2 AS
LANGUAGE JAVA NAME ' EncrUtil. encrypt (java.lang.String) return java.lang.String';
This function can then be used just like any other Oracle function:
SELECT TEST_ENCRYPTOR('Vikram') FROM DUAL;
I was able to move the Java code to Oracle. I am writing this for people who are facing similar issue.
- Log on to server where Oracle database is installed through Putty (command prompt in case of windows)
- Place the Java file in server using WinSCP.
Find the Java compiler present in oracle home using command
find / -name javac
Compile the Java file using Oracle's Java compiler as the Java version used by the database and Java version usually used will be different
$ORACLE_HOME/jdk/bin/javac /home/vikram/EncrUtil.java
Note: Instead of compiling on the database server, a
.class
or.jar
file can be directly loaded into the database. But make sure to use the same version of Java as used by the database.
To find the java version used by oracle
$ORACLE_HOME/jdk/bin/java -version
java version "1.5.0_17"
Java(TM) 2 Runtime Environment, Standard Edition (build 1.5.0_17-b03)
Java HotSpot(TM) 64-Bit Server VM (build 1.5.0_17-b03, mixed mode)
Set ORACLE_HOME if it is not set
export ORACLE_HOME=/database/ora11gr2/product/11.2.0/dbhome_1/
Add
loadjava
's directory in the path (that's the database's bin directory)
export PATH=$PATH:$ORACLE_HOME/bin
Note: In case class not found exception is found go through “loadjava” and check if path present is properly set or variables are defined is not define those
Load the class into the database
loadjava -user <user>/<password>@xxx.xxx.xxx.xxxx:1521:<instance>
-thin –resolve /home/vikram/ EncrUtil.class
-resolve
is used so that.class
file can be resolved before loading into the database as its easy to debug Java outside database.
-thin
is used for thin client
Once the class is loaded, create a wrapper function on top it, so that it can be used in database
CREATE OR REPLACE FUNCTION TEST_DECRYPTOR(indata IN VARCHAR2)
RETURN VARCHAR2 AS
LANGUAGE JAVA NAME ' EncrUtil. decrypt (java.lang.String) return java.lang.String';
CREATE OR REPLACE FUNCTION TEST_ENCRYPTOR (indata IN VARCHAR2)
RETURN VARCHAR2 AS
LANGUAGE JAVA NAME ' EncrUtil. encrypt (java.lang.String) return java.lang.String';
This function can then be used just like any other Oracle function:
SELECT TEST_ENCRYPTOR('Vikram') FROM DUAL;
edited Aug 16 '13 at 7:44


Mat
7,97323338
7,97323338
answered Aug 16 '13 at 7:30
Vikram VVikram V
5614
5614
add a comment |
add a comment |
A little late but the answer may be useful:
In Java this line should be changed:
final IvParameterSpec iv = new IvParameterSpec(new byte[16]);
into this:
final IvParameterSpec iv = new IvParameterSpec(new String(Base64.decode('vector_inicializacion')).getBytes());
where vector_inicializacion is a any hexadecimal of 16 but in PL/SQL it must be the same.
and
strDecryptedText = new String(byteDecryptedText);
Should be replaced by
strDecryptedText = new String(Base64.encodeBytes(byteDecryptedText))
In PL:
encrypted_raw := DBMS_CRYPTO.ENCRYPT
(
--src => UTL_RAW.CAST_TO_RAW (p_plainText),
src =>UTL_I18N.STRING_TO_RAW (p_plainText,'AL32UTF8'),
typ => encryption_type,
key => encryption_key
IV => UTL_RAW.CAST_TO_RAW ('vector_inicializacion')
);
add a comment |
A little late but the answer may be useful:
In Java this line should be changed:
final IvParameterSpec iv = new IvParameterSpec(new byte[16]);
into this:
final IvParameterSpec iv = new IvParameterSpec(new String(Base64.decode('vector_inicializacion')).getBytes());
where vector_inicializacion is a any hexadecimal of 16 but in PL/SQL it must be the same.
and
strDecryptedText = new String(byteDecryptedText);
Should be replaced by
strDecryptedText = new String(Base64.encodeBytes(byteDecryptedText))
In PL:
encrypted_raw := DBMS_CRYPTO.ENCRYPT
(
--src => UTL_RAW.CAST_TO_RAW (p_plainText),
src =>UTL_I18N.STRING_TO_RAW (p_plainText,'AL32UTF8'),
typ => encryption_type,
key => encryption_key
IV => UTL_RAW.CAST_TO_RAW ('vector_inicializacion')
);
add a comment |
A little late but the answer may be useful:
In Java this line should be changed:
final IvParameterSpec iv = new IvParameterSpec(new byte[16]);
into this:
final IvParameterSpec iv = new IvParameterSpec(new String(Base64.decode('vector_inicializacion')).getBytes());
where vector_inicializacion is a any hexadecimal of 16 but in PL/SQL it must be the same.
and
strDecryptedText = new String(byteDecryptedText);
Should be replaced by
strDecryptedText = new String(Base64.encodeBytes(byteDecryptedText))
In PL:
encrypted_raw := DBMS_CRYPTO.ENCRYPT
(
--src => UTL_RAW.CAST_TO_RAW (p_plainText),
src =>UTL_I18N.STRING_TO_RAW (p_plainText,'AL32UTF8'),
typ => encryption_type,
key => encryption_key
IV => UTL_RAW.CAST_TO_RAW ('vector_inicializacion')
);
A little late but the answer may be useful:
In Java this line should be changed:
final IvParameterSpec iv = new IvParameterSpec(new byte[16]);
into this:
final IvParameterSpec iv = new IvParameterSpec(new String(Base64.decode('vector_inicializacion')).getBytes());
where vector_inicializacion is a any hexadecimal of 16 but in PL/SQL it must be the same.
and
strDecryptedText = new String(byteDecryptedText);
Should be replaced by
strDecryptedText = new String(Base64.encodeBytes(byteDecryptedText))
In PL:
encrypted_raw := DBMS_CRYPTO.ENCRYPT
(
--src => UTL_RAW.CAST_TO_RAW (p_plainText),
src =>UTL_I18N.STRING_TO_RAW (p_plainText,'AL32UTF8'),
typ => encryption_type,
key => encryption_key
IV => UTL_RAW.CAST_TO_RAW ('vector_inicializacion')
);
edited Oct 9 '18 at 7:42


Tom V
13.8k74677
13.8k74677
answered Oct 8 '18 at 22:30


Edwin Gauta TorresEdwin Gauta Torres
111
111
add a comment |
add a comment |
ORA-29531: no method encrypt in class EncrUtil
why this error may appear?
New contributor
Oktay Aliyev is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
ORA-29531: no method encrypt in class EncrUtil
why this error may appear?
New contributor
Oktay Aliyev is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
ORA-29531: no method encrypt in class EncrUtil
why this error may appear?
New contributor
Oktay Aliyev is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
ORA-29531: no method encrypt in class EncrUtil
why this error may appear?
New contributor
Oktay Aliyev is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Oktay Aliyev is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 11 mins ago
Oktay AliyevOktay Aliyev
1
1
New contributor
Oktay Aliyev is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Oktay Aliyev is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Oktay Aliyev is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Thanks for contributing an answer to Database Administrators Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fdba.stackexchange.com%2fquestions%2f47696%2freplicate-or-call-java-encryption-function-in-oracle-pl-sql%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
relW3x8APdoSm xNxk2fvDWNzOQPVDWw7,XkIMqbhIEcFvkiZvfLE X