Increment a GUID
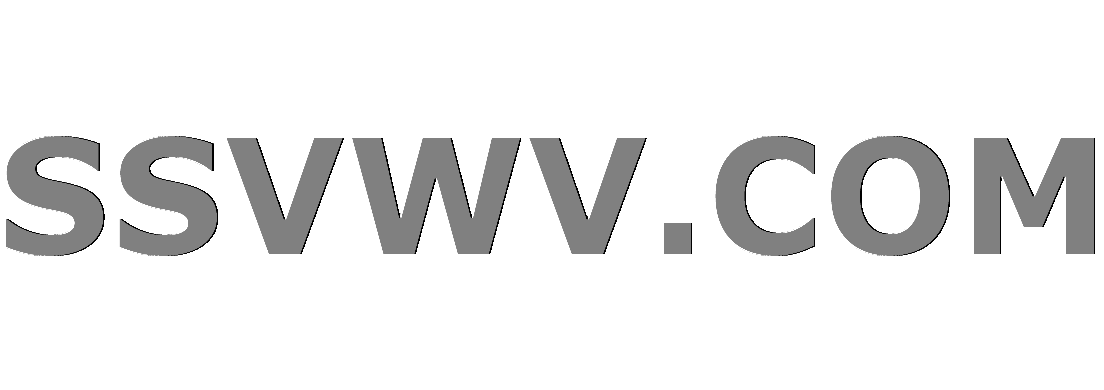
Multi tool use
$begingroup$
Inspired by a recent Daily WTF article...
Write a program or function that takes a GUID (string in the format XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
, where each X represents a hexadecimal digit), and outputs the GUID incremented by one.
Examples
>>> increment_guid('7f128bd4-b0ba-4597-8f35-3a2f2756dfbb')
'7f128bd4-b0ba-4597-8f35-3a2f2756dfbc'
>>> increment_guid('06b86883-f3e7-4f9d-87c5-a047e89a19fa')
'06b86883-f3e7-4f9d-87c5-a047e89a19fb'
>>> increment_guid('89f25f2f-2f7b-4aa6-b9d7-46a98e3cb2cf')
'89f25f2f-2f7b-4aa6-b9d7-46a98e3cb2d0'
>>> increment_guid('8e0f9835-4086-406b-b7a4-532da46963ff')
'8e0f9835-4086-406b-b7a4-532da4696400'
>>> increment_guid('7f128bd4-b0ba-4597-ffff-ffffffffffff')
'7f128bd4-b0ba-4598-0000-000000000000'
Notes
- Unlike in the linked article, incrementing a GUID that ends in F must “carry” to the previous hex digit. See examples above.
- You may assume that the input will not be
ffffffff-ffff-ffff-ffff-ffffffffffff
. - For hex digits above 9, you may use either upper (A-F) or lower (a-f) case.
- Yes, GUIDs may start with a
0
. - Your output must consist of exactly 32 hex digits and 4 hyphens in the expected format, including any necessary leading
0
s.
code-golf
$endgroup$
add a comment |
$begingroup$
Inspired by a recent Daily WTF article...
Write a program or function that takes a GUID (string in the format XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
, where each X represents a hexadecimal digit), and outputs the GUID incremented by one.
Examples
>>> increment_guid('7f128bd4-b0ba-4597-8f35-3a2f2756dfbb')
'7f128bd4-b0ba-4597-8f35-3a2f2756dfbc'
>>> increment_guid('06b86883-f3e7-4f9d-87c5-a047e89a19fa')
'06b86883-f3e7-4f9d-87c5-a047e89a19fb'
>>> increment_guid('89f25f2f-2f7b-4aa6-b9d7-46a98e3cb2cf')
'89f25f2f-2f7b-4aa6-b9d7-46a98e3cb2d0'
>>> increment_guid('8e0f9835-4086-406b-b7a4-532da46963ff')
'8e0f9835-4086-406b-b7a4-532da4696400'
>>> increment_guid('7f128bd4-b0ba-4597-ffff-ffffffffffff')
'7f128bd4-b0ba-4598-0000-000000000000'
Notes
- Unlike in the linked article, incrementing a GUID that ends in F must “carry” to the previous hex digit. See examples above.
- You may assume that the input will not be
ffffffff-ffff-ffff-ffff-ffffffffffff
. - For hex digits above 9, you may use either upper (A-F) or lower (a-f) case.
- Yes, GUIDs may start with a
0
. - Your output must consist of exactly 32 hex digits and 4 hyphens in the expected format, including any necessary leading
0
s.
code-golf
$endgroup$
1
$begingroup$
Are we supposed to leave the 6 fixed bits in the UUIDv4 intact?
$endgroup$
– Filip Haglund
yesterday
2
$begingroup$
@FilipHaglund: No, just treat the GUID as a 128-bit number, where none of the bits have any special meaning. Similarly, GUIDs are assumed to sort in straightforward lexicographical order rather than in the binary order of a WindowsGUID
struct.
$endgroup$
– dan04
yesterday
3
$begingroup$
Suggested test case:89f25f2f-2f7b-4aa6-b9d7-46a98e3cb29f
to ensure that answers can make the transition9 -> a
.
$endgroup$
– Kamil Drakari
yesterday
1
$begingroup$
@dana: You may use any data type for which your language's equivalent of C#'sforeach (char ch in theInput)
is valid.
$endgroup$
– dan04
yesterday
add a comment |
$begingroup$
Inspired by a recent Daily WTF article...
Write a program or function that takes a GUID (string in the format XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
, where each X represents a hexadecimal digit), and outputs the GUID incremented by one.
Examples
>>> increment_guid('7f128bd4-b0ba-4597-8f35-3a2f2756dfbb')
'7f128bd4-b0ba-4597-8f35-3a2f2756dfbc'
>>> increment_guid('06b86883-f3e7-4f9d-87c5-a047e89a19fa')
'06b86883-f3e7-4f9d-87c5-a047e89a19fb'
>>> increment_guid('89f25f2f-2f7b-4aa6-b9d7-46a98e3cb2cf')
'89f25f2f-2f7b-4aa6-b9d7-46a98e3cb2d0'
>>> increment_guid('8e0f9835-4086-406b-b7a4-532da46963ff')
'8e0f9835-4086-406b-b7a4-532da4696400'
>>> increment_guid('7f128bd4-b0ba-4597-ffff-ffffffffffff')
'7f128bd4-b0ba-4598-0000-000000000000'
Notes
- Unlike in the linked article, incrementing a GUID that ends in F must “carry” to the previous hex digit. See examples above.
- You may assume that the input will not be
ffffffff-ffff-ffff-ffff-ffffffffffff
. - For hex digits above 9, you may use either upper (A-F) or lower (a-f) case.
- Yes, GUIDs may start with a
0
. - Your output must consist of exactly 32 hex digits and 4 hyphens in the expected format, including any necessary leading
0
s.
code-golf
$endgroup$
Inspired by a recent Daily WTF article...
Write a program or function that takes a GUID (string in the format XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
, where each X represents a hexadecimal digit), and outputs the GUID incremented by one.
Examples
>>> increment_guid('7f128bd4-b0ba-4597-8f35-3a2f2756dfbb')
'7f128bd4-b0ba-4597-8f35-3a2f2756dfbc'
>>> increment_guid('06b86883-f3e7-4f9d-87c5-a047e89a19fa')
'06b86883-f3e7-4f9d-87c5-a047e89a19fb'
>>> increment_guid('89f25f2f-2f7b-4aa6-b9d7-46a98e3cb2cf')
'89f25f2f-2f7b-4aa6-b9d7-46a98e3cb2d0'
>>> increment_guid('8e0f9835-4086-406b-b7a4-532da46963ff')
'8e0f9835-4086-406b-b7a4-532da4696400'
>>> increment_guid('7f128bd4-b0ba-4597-ffff-ffffffffffff')
'7f128bd4-b0ba-4598-0000-000000000000'
Notes
- Unlike in the linked article, incrementing a GUID that ends in F must “carry” to the previous hex digit. See examples above.
- You may assume that the input will not be
ffffffff-ffff-ffff-ffff-ffffffffffff
. - For hex digits above 9, you may use either upper (A-F) or lower (a-f) case.
- Yes, GUIDs may start with a
0
. - Your output must consist of exactly 32 hex digits and 4 hyphens in the expected format, including any necessary leading
0
s.
code-golf
code-golf
edited yesterday
dan04
asked 2 days ago
dan04dan04
4,12322135
4,12322135
1
$begingroup$
Are we supposed to leave the 6 fixed bits in the UUIDv4 intact?
$endgroup$
– Filip Haglund
yesterday
2
$begingroup$
@FilipHaglund: No, just treat the GUID as a 128-bit number, where none of the bits have any special meaning. Similarly, GUIDs are assumed to sort in straightforward lexicographical order rather than in the binary order of a WindowsGUID
struct.
$endgroup$
– dan04
yesterday
3
$begingroup$
Suggested test case:89f25f2f-2f7b-4aa6-b9d7-46a98e3cb29f
to ensure that answers can make the transition9 -> a
.
$endgroup$
– Kamil Drakari
yesterday
1
$begingroup$
@dana: You may use any data type for which your language's equivalent of C#'sforeach (char ch in theInput)
is valid.
$endgroup$
– dan04
yesterday
add a comment |
1
$begingroup$
Are we supposed to leave the 6 fixed bits in the UUIDv4 intact?
$endgroup$
– Filip Haglund
yesterday
2
$begingroup$
@FilipHaglund: No, just treat the GUID as a 128-bit number, where none of the bits have any special meaning. Similarly, GUIDs are assumed to sort in straightforward lexicographical order rather than in the binary order of a WindowsGUID
struct.
$endgroup$
– dan04
yesterday
3
$begingroup$
Suggested test case:89f25f2f-2f7b-4aa6-b9d7-46a98e3cb29f
to ensure that answers can make the transition9 -> a
.
$endgroup$
– Kamil Drakari
yesterday
1
$begingroup$
@dana: You may use any data type for which your language's equivalent of C#'sforeach (char ch in theInput)
is valid.
$endgroup$
– dan04
yesterday
1
1
$begingroup$
Are we supposed to leave the 6 fixed bits in the UUIDv4 intact?
$endgroup$
– Filip Haglund
yesterday
$begingroup$
Are we supposed to leave the 6 fixed bits in the UUIDv4 intact?
$endgroup$
– Filip Haglund
yesterday
2
2
$begingroup$
@FilipHaglund: No, just treat the GUID as a 128-bit number, where none of the bits have any special meaning. Similarly, GUIDs are assumed to sort in straightforward lexicographical order rather than in the binary order of a Windows
GUID
struct.$endgroup$
– dan04
yesterday
$begingroup$
@FilipHaglund: No, just treat the GUID as a 128-bit number, where none of the bits have any special meaning. Similarly, GUIDs are assumed to sort in straightforward lexicographical order rather than in the binary order of a Windows
GUID
struct.$endgroup$
– dan04
yesterday
3
3
$begingroup$
Suggested test case:
89f25f2f-2f7b-4aa6-b9d7-46a98e3cb29f
to ensure that answers can make the transition 9 -> a
.$endgroup$
– Kamil Drakari
yesterday
$begingroup$
Suggested test case:
89f25f2f-2f7b-4aa6-b9d7-46a98e3cb29f
to ensure that answers can make the transition 9 -> a
.$endgroup$
– Kamil Drakari
yesterday
1
1
$begingroup$
@dana: You may use any data type for which your language's equivalent of C#'s
foreach (char ch in theInput)
is valid.$endgroup$
– dan04
yesterday
$begingroup$
@dana: You may use any data type for which your language's equivalent of C#'s
foreach (char ch in theInput)
is valid.$endgroup$
– dan04
yesterday
add a comment |
18 Answers
18
active
oldest
votes
$begingroup$
Python 2, 50
- 3 bytes saved thanks to @Dennis.
lambda s:UUID(int=UUID(s).int+1)
from uuid import*
Try it online!
$endgroup$
add a comment |
$begingroup$
JavaScript (ES6), 85 bytes
The output string is in lowercase.
s=>(g=(c,x=+('0x'+s[--n])+!!c)=>1/x?g(x>>4)+(x&15).toString(16):~n?g(c)+'-':'')(n=36)
Try it online!
Commented
s => ( // s = GUID
g = ( // g = recursive function taking:
c, // c = carry from the previous iteration
x = +('0x' + s[--n]) // x = decimal conversion of the current digit
+ !!c // add the carry
) => //
1 / x ? // if x is numeric:
g(x >> 4) + // do a recursive call, using the new carry
(x & 15) // and append the next digit
.toString(16) // converted back to hexadecimal
: // else:
~n ? // if n is not equal to -1:
g(c) // do a recursive call, leaving the current carry unchanged
+ '-' // and append a hyphen
: // else:
'' // stop recursion
)(n = 36) // initial call to g with n = 36 and a truthy carry
$endgroup$
add a comment |
$begingroup$
05AB1E, 17 15 18 bytes
Saved 2 bytes thanks to Kevin Cruijssen
'-K1ìH>h¦Ž¦˜S·£'-ý
Try it online!
or as a Test Suite
Explanation
'-K # remove "-" from input
1ì # prepend a 1 (to preserve leading 0s)
H # convert from hex to base 10
> # increment
h # convert to hex from base 10
¦ # remove the extra 1
ަ˜S· # push [8, 4, 4, 4, 12]
£ # split into parts of these sizes
'-ý # join on "-"
$endgroup$
$begingroup$
Dang, you beat me to it.. Had something very similar, but withžKÃ
instead of'-K
. Btw, you can save 2 bytes by changing•É]•S3+
toަ˜S·
.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Thanks! I don't know how many times I've kept forgetting thatŽ
is a thing now...
$endgroup$
– Emigna
yesterday
$begingroup$
I un-accepted this answer because someone pointed out that it will drop leading 0's. Please fix.
$endgroup$
– dan04
yesterday
$begingroup$
@dan04: Good call! I hadn't thought of that. Should be fixed now :)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
APL (Dyalog Unicode), 46 bytesSBCS
Anonymous tacit prefix function.
⎕CY'dfns'
(∊1hex 16(|+1⌽=)⍣≡1+@32dec¨)@('-'≠⊢)
Try it online!
⎕CY'dfns'
copy the "dfns" library (to get hex
and dec
)
(
…)
⊢
the argument
≠
differs from
'-'
a dash(
…)@
on the subset consisting of the locations at which the above criterion is true, apply:
dec¨
convert each hexadecimal character to a decimal number
…@32
at position 32 (the last digit), apply:
1+
increment
16(
…)⍣≡
repeatedly apply with left argument 16 until stable:
=
compare (gives mask where the hexadecimal digits are 16)
1⌽
cyclically rotate one step left (this is the carry bit)
|+
to that, add the division remainder when divided (by sixteen, thus making all 16 into 0)
1hex
turn digits into length-one hexadecimal character representations
∊
ϵnlist (flatten)
$endgroup$
add a comment |
$begingroup$
Java 11, 152 149 111 108 bytes
s->{var b=s.getLeastSignificantBits()+1;return new java.util.UUID(s.getMostSignificantBits()+(b==0?1:0),b);}
-38 bytes thank to @OlivierGrégoire.
-3 bytes thanks to @ASCII-only.
Try it online.
Explanation:
s->{ // Method with UUID as both parameter and return-type
var b=s.getLeastSignificantBits()
// Get the 64 least significant bits of the input-UUID's 128 bits as long
+1; // And increase it by 1
return new java.util.UUID(
// Return a new UUID with:
s.getMostSignificantBits()
// The 64 most significant bits of the input-UUID's 128 bits as long
+(b==0? // And if the 64 least significant bits + 1 are exactly 0:
1 // Increase the 64 most significant bits by 1 as well
: // Else:
0, // Don't change the 64 most significant bits by adding 0
b);} // And the 64 least significant bits + 1
Old 149 bytes answer:
s->{var t=new java.math.BigInteger(s.replace("-",""),16);return(t.add(t.ONE).toString(16)).replaceAll("(.{4})".repeat(5)+"(.*)","$1$2-$3-$4-$5-$6");}
Try it online.
Explanation:
s->{ // Method with String as both parameter and return-type
var t=new java.math.BigInteger( // Create a BigInteger
s.replace("-",""), // Of the input-string with all "-" removed
16); // Converted from Hexadecimal
return(t.add(t.ONE) // Add 1
.toString(16)) // And convert it back to a Hexadecimal String
.replaceAll("(.{4})".repeat(5)+"(.*)",
// And split the string into parts of sizes 4,4,4,4,4,rest
"$1$2-$3-$4-$5-$6");} // And insert "-" after parts of size 8,4,4,4,
// and return it as result
$endgroup$
1
$begingroup$
111 bytes
$endgroup$
– Olivier Grégoire
yesterday
$begingroup$
@OlivierGrégoire Hadn't thought about using an actual UUID! Nice and shorter alternative. :D
$endgroup$
– Kevin Cruijssen
yesterday
1
$begingroup$
109
$endgroup$
– ASCII-only
yesterday
$begingroup$
-1 more with var instead of long
$endgroup$
– ASCII-only
yesterday
add a comment |
$begingroup$
Ruby -pl
, 62 57 55 bytes
$_="%032x"%-~gsub(?-,"").hex
7.step(22,5){|i|$_[i]+=?-}
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 113 112 bytes
def f(s):a=hex(int(s.replace('-',''),16)+1+2**128);return'-'.join((a[3:11],a[11:15],a[15:19],a[19:23],a[23:-1]))
Try it online!
Without imports
$endgroup$
add a comment |
$begingroup$
Retina 0.8.2, 21 bytes
T`FfdlL`0dlL`.[-Ff]*$
Try it online! Link includes test cases. 9
becomes a
. Explanation: The regex matches all trailing f
s and -
s plus one preceding character. The transliteration then cyclically increments those characters as if they were hex digits. Alternate approach, also 21 bytes:
T`L`l
T`fo`dl`.[-f]*$
Try it online! Link includes test cases. Works by lowercasing the input to simplify the transliteration. Would therefore be 15 bytes if it only had to support lowercase. Try it online! Link includes test cases.
$endgroup$
add a comment |
$begingroup$
MATLAB, 138 bytes
a=1;Z=a;for r=flip(split(input(''),'-'))'
q=r{:};z=dec2hex(hex2dec(q)+a,nnz(q));try
z+q;a=0;catch
z=~q+48;end
Z=[z 45 Z];end;disp(Z(1:36))
Fixed a bug in case a chunk is all zeros. Also golfed off a lot by abusing try/catch. Net result: 0 bytes saved.
An attempt to 'cheat' by using java.util.UUID
failed because the long
value returned from java.util.UUID.get[Most/Least]SignificantBits
gets converted to a double
which incurs a loss of precision. I invite you to take a look at this table and silently utter "...but why?"
Explanation
The hex2dec
function spits out a double
, so it cannot process the entire GUID at once to avoid exceeding flintmax
. Instead, we have to process the GUID chunk by chunck, using split
. The variable a
checks if we need to carry a one, and cheatingly also is the initial increment we add. The condition for carrying over is whether the lengths of the original and incremented strings are no longer equal.
Original version was just under 160 bytes so I'd like to think this should not be easy to outgolf.
$endgroup$
add a comment |
$begingroup$
Python 2, 99 bytes
s='%032x'%-~int(input().replace('-',''),16)
print'-'.join((s[:8],s[8:12],s[12:16],s[16:20],s[20:]))
Try it online!
No uuid.UUID
usage.
$endgroup$
add a comment |
$begingroup$
Python 2, 82 bytes
q='f0123456789abcdef--'
f=lambda s:[str,f][s[-1]in'f-'](s[:-1])+q[q.find(s[-1])+1]
Try it online!
No imports or hex conversion.
This scans from the back of the string, moving each character along the cycle 0123456789abcdef
, with -
going to itself. After it hits a symbol other than f
or -
, it stops scanning leftwards, and just returns the remainder unchanged. This solution isn't specific to the UUID format -- any number of blocks of any number of hex letters would work.
The base case of [str,f][s[-1]in'f-'](s[:-1])
is a trick I haven't seen used in a golf before. It terminates the recursion without any if
, and
, or
, or other explicit control flow.
Based on the condition [s[-1]in'f-']
of the last character, the code either returns f(s[:-1])
or just s[:-1]
unchanged. Since str
is the identity on strings, we can select one of the functions [str,f]
and apply it to s[:-1]
. Note that the recursive call with f
is not made if it is not chosen, getting around the common issue problem that Python eagerly evaluates unused options, leading to infinite regress in recursions.
$endgroup$
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 77 bytes
x=>{for(int i=35,c;(x[i]=(char)((c=x[i--])<48?c:c==57?65:c>69?48:c+1))<49;);}
Try it online!
-1 byte thanks to @ASCIIOnly!
Anonymous function that takes a char
as input and outputs by modifying an argument.
Input is scanned from right to left and replaced using the following rules.
- The
-
character is ignored and processing continues - The
F
character is converted to0
and processing continues - The
9
character is converted toA
and processing stops - The characters
A-E
and0-8
are incremented by 1 and processing stops
$endgroup$
2
$begingroup$
==70
->>69
$endgroup$
– ASCII-only
yesterday
$begingroup$
Excellent - Thanks :)
$endgroup$
– dana
yesterday
add a comment |
$begingroup$
Perl 6, 65 bytes
{(:16(TR/-//)+1).base(16).comb.rotor(8,4,4,4,*)».join.join('-')}
Test it
$endgroup$
1
$begingroup$
The OP has clarified that leading zeroes must be preserved.
$endgroup$
– Dennis♦
yesterday
$begingroup$
56 bytes with leading zeroes
$endgroup$
– Jo King
yesterday
1
$begingroup$
Or 53 bytes by handling stuff more manually
$endgroup$
– Jo King
22 hours ago
add a comment |
$begingroup$
Jelly, 20 bytes
-2 (and a bug fix) thanks to Dennis!
ØhiⱮṣ0µẎḅ⁴‘ḃ⁴ịØhṁj”-
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 3, 50 bytes
from uuid import*
lambda u:UUID(int=UUID(u).int+1)
Try it online!
$endgroup$
add a comment |
$begingroup$
JavaScript (Node.js), 81 bytes
s=>s.replace(/.([f-]*)$/,(s,y)=>(('0x'+s[0]|0)+1).toString(16)+y.replace(/f/g,0))
Try it online!
$endgroup$
add a comment |
$begingroup$
PowerShell, 126 bytes
$a=("{0:X32}" -f (1+[Numerics.BigInteger]::Parse($args[0]-replace"-", 'AllowHexSpecifier')));5..2|%{$a=$a.Insert(4*$_,"-")};$a
Try it online!
Pretty trivial answer. Just thought I'd get the beloved PowerShell added to the list :)
$endgroup$
add a comment |
$begingroup$
Perl 5, 64 bytes
$c=reverse((1+hex s/-//gr)->as_hex);$c=~s/..$//;s/[^-]/chop$c/ge
The number of parentheses necessary here makes me sad, but ->
binds very tightly, as ->as_hex
is the quickest way I can find to get hexadecimal-formatted output.
Run with perl -Mbigint -p
. Basically, it just converts the number to a bigint hexadecimal, adds one, and then subtitutes the digits of the result back into the original value, leaving dashes untouched.
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "200"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f178837%2fincrement-a-guid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
18 Answers
18
active
oldest
votes
18 Answers
18
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Python 2, 50
- 3 bytes saved thanks to @Dennis.
lambda s:UUID(int=UUID(s).int+1)
from uuid import*
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 50
- 3 bytes saved thanks to @Dennis.
lambda s:UUID(int=UUID(s).int+1)
from uuid import*
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 50
- 3 bytes saved thanks to @Dennis.
lambda s:UUID(int=UUID(s).int+1)
from uuid import*
Try it online!
$endgroup$
Python 2, 50
- 3 bytes saved thanks to @Dennis.
lambda s:UUID(int=UUID(s).int+1)
from uuid import*
Try it online!
edited 11 hours ago
answered 2 days ago


Digital TraumaDigital Trauma
58.8k787222
58.8k787222
add a comment |
add a comment |
$begingroup$
JavaScript (ES6), 85 bytes
The output string is in lowercase.
s=>(g=(c,x=+('0x'+s[--n])+!!c)=>1/x?g(x>>4)+(x&15).toString(16):~n?g(c)+'-':'')(n=36)
Try it online!
Commented
s => ( // s = GUID
g = ( // g = recursive function taking:
c, // c = carry from the previous iteration
x = +('0x' + s[--n]) // x = decimal conversion of the current digit
+ !!c // add the carry
) => //
1 / x ? // if x is numeric:
g(x >> 4) + // do a recursive call, using the new carry
(x & 15) // and append the next digit
.toString(16) // converted back to hexadecimal
: // else:
~n ? // if n is not equal to -1:
g(c) // do a recursive call, leaving the current carry unchanged
+ '-' // and append a hyphen
: // else:
'' // stop recursion
)(n = 36) // initial call to g with n = 36 and a truthy carry
$endgroup$
add a comment |
$begingroup$
JavaScript (ES6), 85 bytes
The output string is in lowercase.
s=>(g=(c,x=+('0x'+s[--n])+!!c)=>1/x?g(x>>4)+(x&15).toString(16):~n?g(c)+'-':'')(n=36)
Try it online!
Commented
s => ( // s = GUID
g = ( // g = recursive function taking:
c, // c = carry from the previous iteration
x = +('0x' + s[--n]) // x = decimal conversion of the current digit
+ !!c // add the carry
) => //
1 / x ? // if x is numeric:
g(x >> 4) + // do a recursive call, using the new carry
(x & 15) // and append the next digit
.toString(16) // converted back to hexadecimal
: // else:
~n ? // if n is not equal to -1:
g(c) // do a recursive call, leaving the current carry unchanged
+ '-' // and append a hyphen
: // else:
'' // stop recursion
)(n = 36) // initial call to g with n = 36 and a truthy carry
$endgroup$
add a comment |
$begingroup$
JavaScript (ES6), 85 bytes
The output string is in lowercase.
s=>(g=(c,x=+('0x'+s[--n])+!!c)=>1/x?g(x>>4)+(x&15).toString(16):~n?g(c)+'-':'')(n=36)
Try it online!
Commented
s => ( // s = GUID
g = ( // g = recursive function taking:
c, // c = carry from the previous iteration
x = +('0x' + s[--n]) // x = decimal conversion of the current digit
+ !!c // add the carry
) => //
1 / x ? // if x is numeric:
g(x >> 4) + // do a recursive call, using the new carry
(x & 15) // and append the next digit
.toString(16) // converted back to hexadecimal
: // else:
~n ? // if n is not equal to -1:
g(c) // do a recursive call, leaving the current carry unchanged
+ '-' // and append a hyphen
: // else:
'' // stop recursion
)(n = 36) // initial call to g with n = 36 and a truthy carry
$endgroup$
JavaScript (ES6), 85 bytes
The output string is in lowercase.
s=>(g=(c,x=+('0x'+s[--n])+!!c)=>1/x?g(x>>4)+(x&15).toString(16):~n?g(c)+'-':'')(n=36)
Try it online!
Commented
s => ( // s = GUID
g = ( // g = recursive function taking:
c, // c = carry from the previous iteration
x = +('0x' + s[--n]) // x = decimal conversion of the current digit
+ !!c // add the carry
) => //
1 / x ? // if x is numeric:
g(x >> 4) + // do a recursive call, using the new carry
(x & 15) // and append the next digit
.toString(16) // converted back to hexadecimal
: // else:
~n ? // if n is not equal to -1:
g(c) // do a recursive call, leaving the current carry unchanged
+ '-' // and append a hyphen
: // else:
'' // stop recursion
)(n = 36) // initial call to g with n = 36 and a truthy carry
edited yesterday
answered yesterday


ArnauldArnauld
73.5k689309
73.5k689309
add a comment |
add a comment |
$begingroup$
05AB1E, 17 15 18 bytes
Saved 2 bytes thanks to Kevin Cruijssen
'-K1ìH>h¦Ž¦˜S·£'-ý
Try it online!
or as a Test Suite
Explanation
'-K # remove "-" from input
1ì # prepend a 1 (to preserve leading 0s)
H # convert from hex to base 10
> # increment
h # convert to hex from base 10
¦ # remove the extra 1
ަ˜S· # push [8, 4, 4, 4, 12]
£ # split into parts of these sizes
'-ý # join on "-"
$endgroup$
$begingroup$
Dang, you beat me to it.. Had something very similar, but withžKÃ
instead of'-K
. Btw, you can save 2 bytes by changing•É]•S3+
toަ˜S·
.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Thanks! I don't know how many times I've kept forgetting thatŽ
is a thing now...
$endgroup$
– Emigna
yesterday
$begingroup$
I un-accepted this answer because someone pointed out that it will drop leading 0's. Please fix.
$endgroup$
– dan04
yesterday
$begingroup$
@dan04: Good call! I hadn't thought of that. Should be fixed now :)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
05AB1E, 17 15 18 bytes
Saved 2 bytes thanks to Kevin Cruijssen
'-K1ìH>h¦Ž¦˜S·£'-ý
Try it online!
or as a Test Suite
Explanation
'-K # remove "-" from input
1ì # prepend a 1 (to preserve leading 0s)
H # convert from hex to base 10
> # increment
h # convert to hex from base 10
¦ # remove the extra 1
ަ˜S· # push [8, 4, 4, 4, 12]
£ # split into parts of these sizes
'-ý # join on "-"
$endgroup$
$begingroup$
Dang, you beat me to it.. Had something very similar, but withžKÃ
instead of'-K
. Btw, you can save 2 bytes by changing•É]•S3+
toަ˜S·
.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Thanks! I don't know how many times I've kept forgetting thatŽ
is a thing now...
$endgroup$
– Emigna
yesterday
$begingroup$
I un-accepted this answer because someone pointed out that it will drop leading 0's. Please fix.
$endgroup$
– dan04
yesterday
$begingroup$
@dan04: Good call! I hadn't thought of that. Should be fixed now :)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
05AB1E, 17 15 18 bytes
Saved 2 bytes thanks to Kevin Cruijssen
'-K1ìH>h¦Ž¦˜S·£'-ý
Try it online!
or as a Test Suite
Explanation
'-K # remove "-" from input
1ì # prepend a 1 (to preserve leading 0s)
H # convert from hex to base 10
> # increment
h # convert to hex from base 10
¦ # remove the extra 1
ަ˜S· # push [8, 4, 4, 4, 12]
£ # split into parts of these sizes
'-ý # join on "-"
$endgroup$
05AB1E, 17 15 18 bytes
Saved 2 bytes thanks to Kevin Cruijssen
'-K1ìH>h¦Ž¦˜S·£'-ý
Try it online!
or as a Test Suite
Explanation
'-K # remove "-" from input
1ì # prepend a 1 (to preserve leading 0s)
H # convert from hex to base 10
> # increment
h # convert to hex from base 10
¦ # remove the extra 1
ަ˜S· # push [8, 4, 4, 4, 12]
£ # split into parts of these sizes
'-ý # join on "-"
edited yesterday
answered yesterday


EmignaEmigna
45.7k432139
45.7k432139
$begingroup$
Dang, you beat me to it.. Had something very similar, but withžKÃ
instead of'-K
. Btw, you can save 2 bytes by changing•É]•S3+
toަ˜S·
.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Thanks! I don't know how many times I've kept forgetting thatŽ
is a thing now...
$endgroup$
– Emigna
yesterday
$begingroup$
I un-accepted this answer because someone pointed out that it will drop leading 0's. Please fix.
$endgroup$
– dan04
yesterday
$begingroup$
@dan04: Good call! I hadn't thought of that. Should be fixed now :)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
Dang, you beat me to it.. Had something very similar, but withžKÃ
instead of'-K
. Btw, you can save 2 bytes by changing•É]•S3+
toަ˜S·
.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Thanks! I don't know how many times I've kept forgetting thatŽ
is a thing now...
$endgroup$
– Emigna
yesterday
$begingroup$
I un-accepted this answer because someone pointed out that it will drop leading 0's. Please fix.
$endgroup$
– dan04
yesterday
$begingroup$
@dan04: Good call! I hadn't thought of that. Should be fixed now :)
$endgroup$
– Emigna
yesterday
$begingroup$
Dang, you beat me to it.. Had something very similar, but with
žKÃ
instead of '-K
. Btw, you can save 2 bytes by changing •É]•S3+
to ަ˜S·
.$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
Dang, you beat me to it.. Had something very similar, but with
žKÃ
instead of '-K
. Btw, you can save 2 bytes by changing •É]•S3+
to ަ˜S·
.$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Thanks! I don't know how many times I've kept forgetting that
Ž
is a thing now...$endgroup$
– Emigna
yesterday
$begingroup$
@KevinCruijssen: Thanks! I don't know how many times I've kept forgetting that
Ž
is a thing now...$endgroup$
– Emigna
yesterday
$begingroup$
I un-accepted this answer because someone pointed out that it will drop leading 0's. Please fix.
$endgroup$
– dan04
yesterday
$begingroup$
I un-accepted this answer because someone pointed out that it will drop leading 0's. Please fix.
$endgroup$
– dan04
yesterday
$begingroup$
@dan04: Good call! I hadn't thought of that. Should be fixed now :)
$endgroup$
– Emigna
yesterday
$begingroup$
@dan04: Good call! I hadn't thought of that. Should be fixed now :)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
APL (Dyalog Unicode), 46 bytesSBCS
Anonymous tacit prefix function.
⎕CY'dfns'
(∊1hex 16(|+1⌽=)⍣≡1+@32dec¨)@('-'≠⊢)
Try it online!
⎕CY'dfns'
copy the "dfns" library (to get hex
and dec
)
(
…)
⊢
the argument
≠
differs from
'-'
a dash(
…)@
on the subset consisting of the locations at which the above criterion is true, apply:
dec¨
convert each hexadecimal character to a decimal number
…@32
at position 32 (the last digit), apply:
1+
increment
16(
…)⍣≡
repeatedly apply with left argument 16 until stable:
=
compare (gives mask where the hexadecimal digits are 16)
1⌽
cyclically rotate one step left (this is the carry bit)
|+
to that, add the division remainder when divided (by sixteen, thus making all 16 into 0)
1hex
turn digits into length-one hexadecimal character representations
∊
ϵnlist (flatten)
$endgroup$
add a comment |
$begingroup$
APL (Dyalog Unicode), 46 bytesSBCS
Anonymous tacit prefix function.
⎕CY'dfns'
(∊1hex 16(|+1⌽=)⍣≡1+@32dec¨)@('-'≠⊢)
Try it online!
⎕CY'dfns'
copy the "dfns" library (to get hex
and dec
)
(
…)
⊢
the argument
≠
differs from
'-'
a dash(
…)@
on the subset consisting of the locations at which the above criterion is true, apply:
dec¨
convert each hexadecimal character to a decimal number
…@32
at position 32 (the last digit), apply:
1+
increment
16(
…)⍣≡
repeatedly apply with left argument 16 until stable:
=
compare (gives mask where the hexadecimal digits are 16)
1⌽
cyclically rotate one step left (this is the carry bit)
|+
to that, add the division remainder when divided (by sixteen, thus making all 16 into 0)
1hex
turn digits into length-one hexadecimal character representations
∊
ϵnlist (flatten)
$endgroup$
add a comment |
$begingroup$
APL (Dyalog Unicode), 46 bytesSBCS
Anonymous tacit prefix function.
⎕CY'dfns'
(∊1hex 16(|+1⌽=)⍣≡1+@32dec¨)@('-'≠⊢)
Try it online!
⎕CY'dfns'
copy the "dfns" library (to get hex
and dec
)
(
…)
⊢
the argument
≠
differs from
'-'
a dash(
…)@
on the subset consisting of the locations at which the above criterion is true, apply:
dec¨
convert each hexadecimal character to a decimal number
…@32
at position 32 (the last digit), apply:
1+
increment
16(
…)⍣≡
repeatedly apply with left argument 16 until stable:
=
compare (gives mask where the hexadecimal digits are 16)
1⌽
cyclically rotate one step left (this is the carry bit)
|+
to that, add the division remainder when divided (by sixteen, thus making all 16 into 0)
1hex
turn digits into length-one hexadecimal character representations
∊
ϵnlist (flatten)
$endgroup$
APL (Dyalog Unicode), 46 bytesSBCS
Anonymous tacit prefix function.
⎕CY'dfns'
(∊1hex 16(|+1⌽=)⍣≡1+@32dec¨)@('-'≠⊢)
Try it online!
⎕CY'dfns'
copy the "dfns" library (to get hex
and dec
)
(
…)
⊢
the argument
≠
differs from
'-'
a dash(
…)@
on the subset consisting of the locations at which the above criterion is true, apply:
dec¨
convert each hexadecimal character to a decimal number
…@32
at position 32 (the last digit), apply:
1+
increment
16(
…)⍣≡
repeatedly apply with left argument 16 until stable:
=
compare (gives mask where the hexadecimal digits are 16)
1⌽
cyclically rotate one step left (this is the carry bit)
|+
to that, add the division remainder when divided (by sixteen, thus making all 16 into 0)
1hex
turn digits into length-one hexadecimal character representations
∊
ϵnlist (flatten)
edited yesterday
answered yesterday


AdámAdám
29.4k271194
29.4k271194
add a comment |
add a comment |
$begingroup$
Java 11, 152 149 111 108 bytes
s->{var b=s.getLeastSignificantBits()+1;return new java.util.UUID(s.getMostSignificantBits()+(b==0?1:0),b);}
-38 bytes thank to @OlivierGrégoire.
-3 bytes thanks to @ASCII-only.
Try it online.
Explanation:
s->{ // Method with UUID as both parameter and return-type
var b=s.getLeastSignificantBits()
// Get the 64 least significant bits of the input-UUID's 128 bits as long
+1; // And increase it by 1
return new java.util.UUID(
// Return a new UUID with:
s.getMostSignificantBits()
// The 64 most significant bits of the input-UUID's 128 bits as long
+(b==0? // And if the 64 least significant bits + 1 are exactly 0:
1 // Increase the 64 most significant bits by 1 as well
: // Else:
0, // Don't change the 64 most significant bits by adding 0
b);} // And the 64 least significant bits + 1
Old 149 bytes answer:
s->{var t=new java.math.BigInteger(s.replace("-",""),16);return(t.add(t.ONE).toString(16)).replaceAll("(.{4})".repeat(5)+"(.*)","$1$2-$3-$4-$5-$6");}
Try it online.
Explanation:
s->{ // Method with String as both parameter and return-type
var t=new java.math.BigInteger( // Create a BigInteger
s.replace("-",""), // Of the input-string with all "-" removed
16); // Converted from Hexadecimal
return(t.add(t.ONE) // Add 1
.toString(16)) // And convert it back to a Hexadecimal String
.replaceAll("(.{4})".repeat(5)+"(.*)",
// And split the string into parts of sizes 4,4,4,4,4,rest
"$1$2-$3-$4-$5-$6");} // And insert "-" after parts of size 8,4,4,4,
// and return it as result
$endgroup$
1
$begingroup$
111 bytes
$endgroup$
– Olivier Grégoire
yesterday
$begingroup$
@OlivierGrégoire Hadn't thought about using an actual UUID! Nice and shorter alternative. :D
$endgroup$
– Kevin Cruijssen
yesterday
1
$begingroup$
109
$endgroup$
– ASCII-only
yesterday
$begingroup$
-1 more with var instead of long
$endgroup$
– ASCII-only
yesterday
add a comment |
$begingroup$
Java 11, 152 149 111 108 bytes
s->{var b=s.getLeastSignificantBits()+1;return new java.util.UUID(s.getMostSignificantBits()+(b==0?1:0),b);}
-38 bytes thank to @OlivierGrégoire.
-3 bytes thanks to @ASCII-only.
Try it online.
Explanation:
s->{ // Method with UUID as both parameter and return-type
var b=s.getLeastSignificantBits()
// Get the 64 least significant bits of the input-UUID's 128 bits as long
+1; // And increase it by 1
return new java.util.UUID(
// Return a new UUID with:
s.getMostSignificantBits()
// The 64 most significant bits of the input-UUID's 128 bits as long
+(b==0? // And if the 64 least significant bits + 1 are exactly 0:
1 // Increase the 64 most significant bits by 1 as well
: // Else:
0, // Don't change the 64 most significant bits by adding 0
b);} // And the 64 least significant bits + 1
Old 149 bytes answer:
s->{var t=new java.math.BigInteger(s.replace("-",""),16);return(t.add(t.ONE).toString(16)).replaceAll("(.{4})".repeat(5)+"(.*)","$1$2-$3-$4-$5-$6");}
Try it online.
Explanation:
s->{ // Method with String as both parameter and return-type
var t=new java.math.BigInteger( // Create a BigInteger
s.replace("-",""), // Of the input-string with all "-" removed
16); // Converted from Hexadecimal
return(t.add(t.ONE) // Add 1
.toString(16)) // And convert it back to a Hexadecimal String
.replaceAll("(.{4})".repeat(5)+"(.*)",
// And split the string into parts of sizes 4,4,4,4,4,rest
"$1$2-$3-$4-$5-$6");} // And insert "-" after parts of size 8,4,4,4,
// and return it as result
$endgroup$
1
$begingroup$
111 bytes
$endgroup$
– Olivier Grégoire
yesterday
$begingroup$
@OlivierGrégoire Hadn't thought about using an actual UUID! Nice and shorter alternative. :D
$endgroup$
– Kevin Cruijssen
yesterday
1
$begingroup$
109
$endgroup$
– ASCII-only
yesterday
$begingroup$
-1 more with var instead of long
$endgroup$
– ASCII-only
yesterday
add a comment |
$begingroup$
Java 11, 152 149 111 108 bytes
s->{var b=s.getLeastSignificantBits()+1;return new java.util.UUID(s.getMostSignificantBits()+(b==0?1:0),b);}
-38 bytes thank to @OlivierGrégoire.
-3 bytes thanks to @ASCII-only.
Try it online.
Explanation:
s->{ // Method with UUID as both parameter and return-type
var b=s.getLeastSignificantBits()
// Get the 64 least significant bits of the input-UUID's 128 bits as long
+1; // And increase it by 1
return new java.util.UUID(
// Return a new UUID with:
s.getMostSignificantBits()
// The 64 most significant bits of the input-UUID's 128 bits as long
+(b==0? // And if the 64 least significant bits + 1 are exactly 0:
1 // Increase the 64 most significant bits by 1 as well
: // Else:
0, // Don't change the 64 most significant bits by adding 0
b);} // And the 64 least significant bits + 1
Old 149 bytes answer:
s->{var t=new java.math.BigInteger(s.replace("-",""),16);return(t.add(t.ONE).toString(16)).replaceAll("(.{4})".repeat(5)+"(.*)","$1$2-$3-$4-$5-$6");}
Try it online.
Explanation:
s->{ // Method with String as both parameter and return-type
var t=new java.math.BigInteger( // Create a BigInteger
s.replace("-",""), // Of the input-string with all "-" removed
16); // Converted from Hexadecimal
return(t.add(t.ONE) // Add 1
.toString(16)) // And convert it back to a Hexadecimal String
.replaceAll("(.{4})".repeat(5)+"(.*)",
// And split the string into parts of sizes 4,4,4,4,4,rest
"$1$2-$3-$4-$5-$6");} // And insert "-" after parts of size 8,4,4,4,
// and return it as result
$endgroup$
Java 11, 152 149 111 108 bytes
s->{var b=s.getLeastSignificantBits()+1;return new java.util.UUID(s.getMostSignificantBits()+(b==0?1:0),b);}
-38 bytes thank to @OlivierGrégoire.
-3 bytes thanks to @ASCII-only.
Try it online.
Explanation:
s->{ // Method with UUID as both parameter and return-type
var b=s.getLeastSignificantBits()
// Get the 64 least significant bits of the input-UUID's 128 bits as long
+1; // And increase it by 1
return new java.util.UUID(
// Return a new UUID with:
s.getMostSignificantBits()
// The 64 most significant bits of the input-UUID's 128 bits as long
+(b==0? // And if the 64 least significant bits + 1 are exactly 0:
1 // Increase the 64 most significant bits by 1 as well
: // Else:
0, // Don't change the 64 most significant bits by adding 0
b);} // And the 64 least significant bits + 1
Old 149 bytes answer:
s->{var t=new java.math.BigInteger(s.replace("-",""),16);return(t.add(t.ONE).toString(16)).replaceAll("(.{4})".repeat(5)+"(.*)","$1$2-$3-$4-$5-$6");}
Try it online.
Explanation:
s->{ // Method with String as both parameter and return-type
var t=new java.math.BigInteger( // Create a BigInteger
s.replace("-",""), // Of the input-string with all "-" removed
16); // Converted from Hexadecimal
return(t.add(t.ONE) // Add 1
.toString(16)) // And convert it back to a Hexadecimal String
.replaceAll("(.{4})".repeat(5)+"(.*)",
// And split the string into parts of sizes 4,4,4,4,4,rest
"$1$2-$3-$4-$5-$6");} // And insert "-" after parts of size 8,4,4,4,
// and return it as result
edited 23 hours ago
answered yesterday


Kevin CruijssenKevin Cruijssen
36.5k555192
36.5k555192
1
$begingroup$
111 bytes
$endgroup$
– Olivier Grégoire
yesterday
$begingroup$
@OlivierGrégoire Hadn't thought about using an actual UUID! Nice and shorter alternative. :D
$endgroup$
– Kevin Cruijssen
yesterday
1
$begingroup$
109
$endgroup$
– ASCII-only
yesterday
$begingroup$
-1 more with var instead of long
$endgroup$
– ASCII-only
yesterday
add a comment |
1
$begingroup$
111 bytes
$endgroup$
– Olivier Grégoire
yesterday
$begingroup$
@OlivierGrégoire Hadn't thought about using an actual UUID! Nice and shorter alternative. :D
$endgroup$
– Kevin Cruijssen
yesterday
1
$begingroup$
109
$endgroup$
– ASCII-only
yesterday
$begingroup$
-1 more with var instead of long
$endgroup$
– ASCII-only
yesterday
1
1
$begingroup$
111 bytes
$endgroup$
– Olivier Grégoire
yesterday
$begingroup$
111 bytes
$endgroup$
– Olivier Grégoire
yesterday
$begingroup$
@OlivierGrégoire Hadn't thought about using an actual UUID! Nice and shorter alternative. :D
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@OlivierGrégoire Hadn't thought about using an actual UUID! Nice and shorter alternative. :D
$endgroup$
– Kevin Cruijssen
yesterday
1
1
$begingroup$
109
$endgroup$
– ASCII-only
yesterday
$begingroup$
109
$endgroup$
– ASCII-only
yesterday
$begingroup$
-1 more with var instead of long
$endgroup$
– ASCII-only
yesterday
$begingroup$
-1 more with var instead of long
$endgroup$
– ASCII-only
yesterday
add a comment |
$begingroup$
Ruby -pl
, 62 57 55 bytes
$_="%032x"%-~gsub(?-,"").hex
7.step(22,5){|i|$_[i]+=?-}
Try it online!
$endgroup$
add a comment |
$begingroup$
Ruby -pl
, 62 57 55 bytes
$_="%032x"%-~gsub(?-,"").hex
7.step(22,5){|i|$_[i]+=?-}
Try it online!
$endgroup$
add a comment |
$begingroup$
Ruby -pl
, 62 57 55 bytes
$_="%032x"%-~gsub(?-,"").hex
7.step(22,5){|i|$_[i]+=?-}
Try it online!
$endgroup$
Ruby -pl
, 62 57 55 bytes
$_="%032x"%-~gsub(?-,"").hex
7.step(22,5){|i|$_[i]+=?-}
Try it online!
edited 22 hours ago
answered yesterday
Kirill L.Kirill L.
3,8051319
3,8051319
add a comment |
add a comment |
$begingroup$
Python 2, 113 112 bytes
def f(s):a=hex(int(s.replace('-',''),16)+1+2**128);return'-'.join((a[3:11],a[11:15],a[15:19],a[19:23],a[23:-1]))
Try it online!
Without imports
$endgroup$
add a comment |
$begingroup$
Python 2, 113 112 bytes
def f(s):a=hex(int(s.replace('-',''),16)+1+2**128);return'-'.join((a[3:11],a[11:15],a[15:19],a[19:23],a[23:-1]))
Try it online!
Without imports
$endgroup$
add a comment |
$begingroup$
Python 2, 113 112 bytes
def f(s):a=hex(int(s.replace('-',''),16)+1+2**128);return'-'.join((a[3:11],a[11:15],a[15:19],a[19:23],a[23:-1]))
Try it online!
Without imports
$endgroup$
Python 2, 113 112 bytes
def f(s):a=hex(int(s.replace('-',''),16)+1+2**128);return'-'.join((a[3:11],a[11:15],a[15:19],a[19:23],a[23:-1]))
Try it online!
Without imports
edited yesterday
answered yesterday


TFeldTFeld
14.6k21241
14.6k21241
add a comment |
add a comment |
$begingroup$
Retina 0.8.2, 21 bytes
T`FfdlL`0dlL`.[-Ff]*$
Try it online! Link includes test cases. 9
becomes a
. Explanation: The regex matches all trailing f
s and -
s plus one preceding character. The transliteration then cyclically increments those characters as if they were hex digits. Alternate approach, also 21 bytes:
T`L`l
T`fo`dl`.[-f]*$
Try it online! Link includes test cases. Works by lowercasing the input to simplify the transliteration. Would therefore be 15 bytes if it only had to support lowercase. Try it online! Link includes test cases.
$endgroup$
add a comment |
$begingroup$
Retina 0.8.2, 21 bytes
T`FfdlL`0dlL`.[-Ff]*$
Try it online! Link includes test cases. 9
becomes a
. Explanation: The regex matches all trailing f
s and -
s plus one preceding character. The transliteration then cyclically increments those characters as if they were hex digits. Alternate approach, also 21 bytes:
T`L`l
T`fo`dl`.[-f]*$
Try it online! Link includes test cases. Works by lowercasing the input to simplify the transliteration. Would therefore be 15 bytes if it only had to support lowercase. Try it online! Link includes test cases.
$endgroup$
add a comment |
$begingroup$
Retina 0.8.2, 21 bytes
T`FfdlL`0dlL`.[-Ff]*$
Try it online! Link includes test cases. 9
becomes a
. Explanation: The regex matches all trailing f
s and -
s plus one preceding character. The transliteration then cyclically increments those characters as if they were hex digits. Alternate approach, also 21 bytes:
T`L`l
T`fo`dl`.[-f]*$
Try it online! Link includes test cases. Works by lowercasing the input to simplify the transliteration. Would therefore be 15 bytes if it only had to support lowercase. Try it online! Link includes test cases.
$endgroup$
Retina 0.8.2, 21 bytes
T`FfdlL`0dlL`.[-Ff]*$
Try it online! Link includes test cases. 9
becomes a
. Explanation: The regex matches all trailing f
s and -
s plus one preceding character. The transliteration then cyclically increments those characters as if they were hex digits. Alternate approach, also 21 bytes:
T`L`l
T`fo`dl`.[-f]*$
Try it online! Link includes test cases. Works by lowercasing the input to simplify the transliteration. Would therefore be 15 bytes if it only had to support lowercase. Try it online! Link includes test cases.
answered yesterday
NeilNeil
79.9k744177
79.9k744177
add a comment |
add a comment |
$begingroup$
MATLAB, 138 bytes
a=1;Z=a;for r=flip(split(input(''),'-'))'
q=r{:};z=dec2hex(hex2dec(q)+a,nnz(q));try
z+q;a=0;catch
z=~q+48;end
Z=[z 45 Z];end;disp(Z(1:36))
Fixed a bug in case a chunk is all zeros. Also golfed off a lot by abusing try/catch. Net result: 0 bytes saved.
An attempt to 'cheat' by using java.util.UUID
failed because the long
value returned from java.util.UUID.get[Most/Least]SignificantBits
gets converted to a double
which incurs a loss of precision. I invite you to take a look at this table and silently utter "...but why?"
Explanation
The hex2dec
function spits out a double
, so it cannot process the entire GUID at once to avoid exceeding flintmax
. Instead, we have to process the GUID chunk by chunck, using split
. The variable a
checks if we need to carry a one, and cheatingly also is the initial increment we add. The condition for carrying over is whether the lengths of the original and incremented strings are no longer equal.
Original version was just under 160 bytes so I'd like to think this should not be easy to outgolf.
$endgroup$
add a comment |
$begingroup$
MATLAB, 138 bytes
a=1;Z=a;for r=flip(split(input(''),'-'))'
q=r{:};z=dec2hex(hex2dec(q)+a,nnz(q));try
z+q;a=0;catch
z=~q+48;end
Z=[z 45 Z];end;disp(Z(1:36))
Fixed a bug in case a chunk is all zeros. Also golfed off a lot by abusing try/catch. Net result: 0 bytes saved.
An attempt to 'cheat' by using java.util.UUID
failed because the long
value returned from java.util.UUID.get[Most/Least]SignificantBits
gets converted to a double
which incurs a loss of precision. I invite you to take a look at this table and silently utter "...but why?"
Explanation
The hex2dec
function spits out a double
, so it cannot process the entire GUID at once to avoid exceeding flintmax
. Instead, we have to process the GUID chunk by chunck, using split
. The variable a
checks if we need to carry a one, and cheatingly also is the initial increment we add. The condition for carrying over is whether the lengths of the original and incremented strings are no longer equal.
Original version was just under 160 bytes so I'd like to think this should not be easy to outgolf.
$endgroup$
add a comment |
$begingroup$
MATLAB, 138 bytes
a=1;Z=a;for r=flip(split(input(''),'-'))'
q=r{:};z=dec2hex(hex2dec(q)+a,nnz(q));try
z+q;a=0;catch
z=~q+48;end
Z=[z 45 Z];end;disp(Z(1:36))
Fixed a bug in case a chunk is all zeros. Also golfed off a lot by abusing try/catch. Net result: 0 bytes saved.
An attempt to 'cheat' by using java.util.UUID
failed because the long
value returned from java.util.UUID.get[Most/Least]SignificantBits
gets converted to a double
which incurs a loss of precision. I invite you to take a look at this table and silently utter "...but why?"
Explanation
The hex2dec
function spits out a double
, so it cannot process the entire GUID at once to avoid exceeding flintmax
. Instead, we have to process the GUID chunk by chunck, using split
. The variable a
checks if we need to carry a one, and cheatingly also is the initial increment we add. The condition for carrying over is whether the lengths of the original and incremented strings are no longer equal.
Original version was just under 160 bytes so I'd like to think this should not be easy to outgolf.
$endgroup$
MATLAB, 138 bytes
a=1;Z=a;for r=flip(split(input(''),'-'))'
q=r{:};z=dec2hex(hex2dec(q)+a,nnz(q));try
z+q;a=0;catch
z=~q+48;end
Z=[z 45 Z];end;disp(Z(1:36))
Fixed a bug in case a chunk is all zeros. Also golfed off a lot by abusing try/catch. Net result: 0 bytes saved.
An attempt to 'cheat' by using java.util.UUID
failed because the long
value returned from java.util.UUID.get[Most/Least]SignificantBits
gets converted to a double
which incurs a loss of precision. I invite you to take a look at this table and silently utter "...but why?"
Explanation
The hex2dec
function spits out a double
, so it cannot process the entire GUID at once to avoid exceeding flintmax
. Instead, we have to process the GUID chunk by chunck, using split
. The variable a
checks if we need to carry a one, and cheatingly also is the initial increment we add. The condition for carrying over is whether the lengths of the original and incremented strings are no longer equal.
Original version was just under 160 bytes so I'd like to think this should not be easy to outgolf.
edited yesterday
answered yesterday


SanchisesSanchises
5,78212351
5,78212351
add a comment |
add a comment |
$begingroup$
Python 2, 99 bytes
s='%032x'%-~int(input().replace('-',''),16)
print'-'.join((s[:8],s[8:12],s[12:16],s[16:20],s[20:]))
Try it online!
No uuid.UUID
usage.
$endgroup$
add a comment |
$begingroup$
Python 2, 99 bytes
s='%032x'%-~int(input().replace('-',''),16)
print'-'.join((s[:8],s[8:12],s[12:16],s[16:20],s[20:]))
Try it online!
No uuid.UUID
usage.
$endgroup$
add a comment |
$begingroup$
Python 2, 99 bytes
s='%032x'%-~int(input().replace('-',''),16)
print'-'.join((s[:8],s[8:12],s[12:16],s[16:20],s[20:]))
Try it online!
No uuid.UUID
usage.
$endgroup$
Python 2, 99 bytes
s='%032x'%-~int(input().replace('-',''),16)
print'-'.join((s[:8],s[8:12],s[12:16],s[16:20],s[20:]))
Try it online!
No uuid.UUID
usage.
answered yesterday


Erik the OutgolferErik the Outgolfer
31.6k429103
31.6k429103
add a comment |
add a comment |
$begingroup$
Python 2, 82 bytes
q='f0123456789abcdef--'
f=lambda s:[str,f][s[-1]in'f-'](s[:-1])+q[q.find(s[-1])+1]
Try it online!
No imports or hex conversion.
This scans from the back of the string, moving each character along the cycle 0123456789abcdef
, with -
going to itself. After it hits a symbol other than f
or -
, it stops scanning leftwards, and just returns the remainder unchanged. This solution isn't specific to the UUID format -- any number of blocks of any number of hex letters would work.
The base case of [str,f][s[-1]in'f-'](s[:-1])
is a trick I haven't seen used in a golf before. It terminates the recursion without any if
, and
, or
, or other explicit control flow.
Based on the condition [s[-1]in'f-']
of the last character, the code either returns f(s[:-1])
or just s[:-1]
unchanged. Since str
is the identity on strings, we can select one of the functions [str,f]
and apply it to s[:-1]
. Note that the recursive call with f
is not made if it is not chosen, getting around the common issue problem that Python eagerly evaluates unused options, leading to infinite regress in recursions.
$endgroup$
add a comment |
$begingroup$
Python 2, 82 bytes
q='f0123456789abcdef--'
f=lambda s:[str,f][s[-1]in'f-'](s[:-1])+q[q.find(s[-1])+1]
Try it online!
No imports or hex conversion.
This scans from the back of the string, moving each character along the cycle 0123456789abcdef
, with -
going to itself. After it hits a symbol other than f
or -
, it stops scanning leftwards, and just returns the remainder unchanged. This solution isn't specific to the UUID format -- any number of blocks of any number of hex letters would work.
The base case of [str,f][s[-1]in'f-'](s[:-1])
is a trick I haven't seen used in a golf before. It terminates the recursion without any if
, and
, or
, or other explicit control flow.
Based on the condition [s[-1]in'f-']
of the last character, the code either returns f(s[:-1])
or just s[:-1]
unchanged. Since str
is the identity on strings, we can select one of the functions [str,f]
and apply it to s[:-1]
. Note that the recursive call with f
is not made if it is not chosen, getting around the common issue problem that Python eagerly evaluates unused options, leading to infinite regress in recursions.
$endgroup$
add a comment |
$begingroup$
Python 2, 82 bytes
q='f0123456789abcdef--'
f=lambda s:[str,f][s[-1]in'f-'](s[:-1])+q[q.find(s[-1])+1]
Try it online!
No imports or hex conversion.
This scans from the back of the string, moving each character along the cycle 0123456789abcdef
, with -
going to itself. After it hits a symbol other than f
or -
, it stops scanning leftwards, and just returns the remainder unchanged. This solution isn't specific to the UUID format -- any number of blocks of any number of hex letters would work.
The base case of [str,f][s[-1]in'f-'](s[:-1])
is a trick I haven't seen used in a golf before. It terminates the recursion without any if
, and
, or
, or other explicit control flow.
Based on the condition [s[-1]in'f-']
of the last character, the code either returns f(s[:-1])
or just s[:-1]
unchanged. Since str
is the identity on strings, we can select one of the functions [str,f]
and apply it to s[:-1]
. Note that the recursive call with f
is not made if it is not chosen, getting around the common issue problem that Python eagerly evaluates unused options, leading to infinite regress in recursions.
$endgroup$
Python 2, 82 bytes
q='f0123456789abcdef--'
f=lambda s:[str,f][s[-1]in'f-'](s[:-1])+q[q.find(s[-1])+1]
Try it online!
No imports or hex conversion.
This scans from the back of the string, moving each character along the cycle 0123456789abcdef
, with -
going to itself. After it hits a symbol other than f
or -
, it stops scanning leftwards, and just returns the remainder unchanged. This solution isn't specific to the UUID format -- any number of blocks of any number of hex letters would work.
The base case of [str,f][s[-1]in'f-'](s[:-1])
is a trick I haven't seen used in a golf before. It terminates the recursion without any if
, and
, or
, or other explicit control flow.
Based on the condition [s[-1]in'f-']
of the last character, the code either returns f(s[:-1])
or just s[:-1]
unchanged. Since str
is the identity on strings, we can select one of the functions [str,f]
and apply it to s[:-1]
. Note that the recursive call with f
is not made if it is not chosen, getting around the common issue problem that Python eagerly evaluates unused options, leading to infinite regress in recursions.
edited yesterday
answered yesterday


xnorxnor
89.9k18185439
89.9k18185439
add a comment |
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 77 bytes
x=>{for(int i=35,c;(x[i]=(char)((c=x[i--])<48?c:c==57?65:c>69?48:c+1))<49;);}
Try it online!
-1 byte thanks to @ASCIIOnly!
Anonymous function that takes a char
as input and outputs by modifying an argument.
Input is scanned from right to left and replaced using the following rules.
- The
-
character is ignored and processing continues - The
F
character is converted to0
and processing continues - The
9
character is converted toA
and processing stops - The characters
A-E
and0-8
are incremented by 1 and processing stops
$endgroup$
2
$begingroup$
==70
->>69
$endgroup$
– ASCII-only
yesterday
$begingroup$
Excellent - Thanks :)
$endgroup$
– dana
yesterday
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 77 bytes
x=>{for(int i=35,c;(x[i]=(char)((c=x[i--])<48?c:c==57?65:c>69?48:c+1))<49;);}
Try it online!
-1 byte thanks to @ASCIIOnly!
Anonymous function that takes a char
as input and outputs by modifying an argument.
Input is scanned from right to left and replaced using the following rules.
- The
-
character is ignored and processing continues - The
F
character is converted to0
and processing continues - The
9
character is converted toA
and processing stops - The characters
A-E
and0-8
are incremented by 1 and processing stops
$endgroup$
2
$begingroup$
==70
->>69
$endgroup$
– ASCII-only
yesterday
$begingroup$
Excellent - Thanks :)
$endgroup$
– dana
yesterday
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 77 bytes
x=>{for(int i=35,c;(x[i]=(char)((c=x[i--])<48?c:c==57?65:c>69?48:c+1))<49;);}
Try it online!
-1 byte thanks to @ASCIIOnly!
Anonymous function that takes a char
as input and outputs by modifying an argument.
Input is scanned from right to left and replaced using the following rules.
- The
-
character is ignored and processing continues - The
F
character is converted to0
and processing continues - The
9
character is converted toA
and processing stops - The characters
A-E
and0-8
are incremented by 1 and processing stops
$endgroup$
C# (Visual C# Interactive Compiler), 77 bytes
x=>{for(int i=35,c;(x[i]=(char)((c=x[i--])<48?c:c==57?65:c>69?48:c+1))<49;);}
Try it online!
-1 byte thanks to @ASCIIOnly!
Anonymous function that takes a char
as input and outputs by modifying an argument.
Input is scanned from right to left and replaced using the following rules.
- The
-
character is ignored and processing continues - The
F
character is converted to0
and processing continues - The
9
character is converted toA
and processing stops - The characters
A-E
and0-8
are incremented by 1 and processing stops
edited yesterday
answered yesterday


danadana
67145
67145
2
$begingroup$
==70
->>69
$endgroup$
– ASCII-only
yesterday
$begingroup$
Excellent - Thanks :)
$endgroup$
– dana
yesterday
add a comment |
2
$begingroup$
==70
->>69
$endgroup$
– ASCII-only
yesterday
$begingroup$
Excellent - Thanks :)
$endgroup$
– dana
yesterday
2
2
$begingroup$
==70
-> >69
$endgroup$
– ASCII-only
yesterday
$begingroup$
==70
-> >69
$endgroup$
– ASCII-only
yesterday
$begingroup$
Excellent - Thanks :)
$endgroup$
– dana
yesterday
$begingroup$
Excellent - Thanks :)
$endgroup$
– dana
yesterday
add a comment |
$begingroup$
Perl 6, 65 bytes
{(:16(TR/-//)+1).base(16).comb.rotor(8,4,4,4,*)».join.join('-')}
Test it
$endgroup$
1
$begingroup$
The OP has clarified that leading zeroes must be preserved.
$endgroup$
– Dennis♦
yesterday
$begingroup$
56 bytes with leading zeroes
$endgroup$
– Jo King
yesterday
1
$begingroup$
Or 53 bytes by handling stuff more manually
$endgroup$
– Jo King
22 hours ago
add a comment |
$begingroup$
Perl 6, 65 bytes
{(:16(TR/-//)+1).base(16).comb.rotor(8,4,4,4,*)».join.join('-')}
Test it
$endgroup$
1
$begingroup$
The OP has clarified that leading zeroes must be preserved.
$endgroup$
– Dennis♦
yesterday
$begingroup$
56 bytes with leading zeroes
$endgroup$
– Jo King
yesterday
1
$begingroup$
Or 53 bytes by handling stuff more manually
$endgroup$
– Jo King
22 hours ago
add a comment |
$begingroup$
Perl 6, 65 bytes
{(:16(TR/-//)+1).base(16).comb.rotor(8,4,4,4,*)».join.join('-')}
Test it
$endgroup$
Perl 6, 65 bytes
{(:16(TR/-//)+1).base(16).comb.rotor(8,4,4,4,*)».join.join('-')}
Test it
answered 2 days ago
Brad Gilbert b2gillsBrad Gilbert b2gills
12.2k11232
12.2k11232
1
$begingroup$
The OP has clarified that leading zeroes must be preserved.
$endgroup$
– Dennis♦
yesterday
$begingroup$
56 bytes with leading zeroes
$endgroup$
– Jo King
yesterday
1
$begingroup$
Or 53 bytes by handling stuff more manually
$endgroup$
– Jo King
22 hours ago
add a comment |
1
$begingroup$
The OP has clarified that leading zeroes must be preserved.
$endgroup$
– Dennis♦
yesterday
$begingroup$
56 bytes with leading zeroes
$endgroup$
– Jo King
yesterday
1
$begingroup$
Or 53 bytes by handling stuff more manually
$endgroup$
– Jo King
22 hours ago
1
1
$begingroup$
The OP has clarified that leading zeroes must be preserved.
$endgroup$
– Dennis♦
yesterday
$begingroup$
The OP has clarified that leading zeroes must be preserved.
$endgroup$
– Dennis♦
yesterday
$begingroup$
56 bytes with leading zeroes
$endgroup$
– Jo King
yesterday
$begingroup$
56 bytes with leading zeroes
$endgroup$
– Jo King
yesterday
1
1
$begingroup$
Or 53 bytes by handling stuff more manually
$endgroup$
– Jo King
22 hours ago
$begingroup$
Or 53 bytes by handling stuff more manually
$endgroup$
– Jo King
22 hours ago
add a comment |
$begingroup$
Jelly, 20 bytes
-2 (and a bug fix) thanks to Dennis!
ØhiⱮṣ0µẎḅ⁴‘ḃ⁴ịØhṁj”-
Try it online!
$endgroup$
add a comment |
$begingroup$
Jelly, 20 bytes
-2 (and a bug fix) thanks to Dennis!
ØhiⱮṣ0µẎḅ⁴‘ḃ⁴ịØhṁj”-
Try it online!
$endgroup$
add a comment |
$begingroup$
Jelly, 20 bytes
-2 (and a bug fix) thanks to Dennis!
ØhiⱮṣ0µẎḅ⁴‘ḃ⁴ịØhṁj”-
Try it online!
$endgroup$
Jelly, 20 bytes
-2 (and a bug fix) thanks to Dennis!
ØhiⱮṣ0µẎḅ⁴‘ḃ⁴ịØhṁj”-
Try it online!
edited 12 hours ago
answered yesterday


Jonathan AllanJonathan Allan
51.2k534166
51.2k534166
add a comment |
add a comment |
$begingroup$
Python 3, 50 bytes
from uuid import*
lambda u:UUID(int=UUID(u).int+1)
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 3, 50 bytes
from uuid import*
lambda u:UUID(int=UUID(u).int+1)
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 3, 50 bytes
from uuid import*
lambda u:UUID(int=UUID(u).int+1)
Try it online!
$endgroup$
Python 3, 50 bytes
from uuid import*
lambda u:UUID(int=UUID(u).int+1)
Try it online!
answered 11 hours ago
DraconisDraconis
411310
411310
add a comment |
add a comment |
$begingroup$
JavaScript (Node.js), 81 bytes
s=>s.replace(/.([f-]*)$/,(s,y)=>(('0x'+s[0]|0)+1).toString(16)+y.replace(/f/g,0))
Try it online!
$endgroup$
add a comment |
$begingroup$
JavaScript (Node.js), 81 bytes
s=>s.replace(/.([f-]*)$/,(s,y)=>(('0x'+s[0]|0)+1).toString(16)+y.replace(/f/g,0))
Try it online!
$endgroup$
add a comment |
$begingroup$
JavaScript (Node.js), 81 bytes
s=>s.replace(/.([f-]*)$/,(s,y)=>(('0x'+s[0]|0)+1).toString(16)+y.replace(/f/g,0))
Try it online!
$endgroup$
JavaScript (Node.js), 81 bytes
s=>s.replace(/.([f-]*)$/,(s,y)=>(('0x'+s[0]|0)+1).toString(16)+y.replace(/f/g,0))
Try it online!
answered yesterday
tshtsh
8,54511547
8,54511547
add a comment |
add a comment |
$begingroup$
PowerShell, 126 bytes
$a=("{0:X32}" -f (1+[Numerics.BigInteger]::Parse($args[0]-replace"-", 'AllowHexSpecifier')));5..2|%{$a=$a.Insert(4*$_,"-")};$a
Try it online!
Pretty trivial answer. Just thought I'd get the beloved PowerShell added to the list :)
$endgroup$
add a comment |
$begingroup$
PowerShell, 126 bytes
$a=("{0:X32}" -f (1+[Numerics.BigInteger]::Parse($args[0]-replace"-", 'AllowHexSpecifier')));5..2|%{$a=$a.Insert(4*$_,"-")};$a
Try it online!
Pretty trivial answer. Just thought I'd get the beloved PowerShell added to the list :)
$endgroup$
add a comment |
$begingroup$
PowerShell, 126 bytes
$a=("{0:X32}" -f (1+[Numerics.BigInteger]::Parse($args[0]-replace"-", 'AllowHexSpecifier')));5..2|%{$a=$a.Insert(4*$_,"-")};$a
Try it online!
Pretty trivial answer. Just thought I'd get the beloved PowerShell added to the list :)
$endgroup$
PowerShell, 126 bytes
$a=("{0:X32}" -f (1+[Numerics.BigInteger]::Parse($args[0]-replace"-", 'AllowHexSpecifier')));5..2|%{$a=$a.Insert(4*$_,"-")};$a
Try it online!
Pretty trivial answer. Just thought I'd get the beloved PowerShell added to the list :)
answered 8 hours ago


KGlasierKGlasier
1316
1316
add a comment |
add a comment |
$begingroup$
Perl 5, 64 bytes
$c=reverse((1+hex s/-//gr)->as_hex);$c=~s/..$//;s/[^-]/chop$c/ge
The number of parentheses necessary here makes me sad, but ->
binds very tightly, as ->as_hex
is the quickest way I can find to get hexadecimal-formatted output.
Run with perl -Mbigint -p
. Basically, it just converts the number to a bigint hexadecimal, adds one, and then subtitutes the digits of the result back into the original value, leaving dashes untouched.
$endgroup$
add a comment |
$begingroup$
Perl 5, 64 bytes
$c=reverse((1+hex s/-//gr)->as_hex);$c=~s/..$//;s/[^-]/chop$c/ge
The number of parentheses necessary here makes me sad, but ->
binds very tightly, as ->as_hex
is the quickest way I can find to get hexadecimal-formatted output.
Run with perl -Mbigint -p
. Basically, it just converts the number to a bigint hexadecimal, adds one, and then subtitutes the digits of the result back into the original value, leaving dashes untouched.
$endgroup$
add a comment |
$begingroup$
Perl 5, 64 bytes
$c=reverse((1+hex s/-//gr)->as_hex);$c=~s/..$//;s/[^-]/chop$c/ge
The number of parentheses necessary here makes me sad, but ->
binds very tightly, as ->as_hex
is the quickest way I can find to get hexadecimal-formatted output.
Run with perl -Mbigint -p
. Basically, it just converts the number to a bigint hexadecimal, adds one, and then subtitutes the digits of the result back into the original value, leaving dashes untouched.
$endgroup$
Perl 5, 64 bytes
$c=reverse((1+hex s/-//gr)->as_hex);$c=~s/..$//;s/[^-]/chop$c/ge
The number of parentheses necessary here makes me sad, but ->
binds very tightly, as ->as_hex
is the quickest way I can find to get hexadecimal-formatted output.
Run with perl -Mbigint -p
. Basically, it just converts the number to a bigint hexadecimal, adds one, and then subtitutes the digits of the result back into the original value, leaving dashes untouched.
answered 4 hours ago


Silvio MayoloSilvio Mayolo
1,407917
1,407917
add a comment |
add a comment |
If this is an answer to a challenge…
…Be sure to follow the challenge specification. However, please refrain from exploiting obvious loopholes. Answers abusing any of the standard loopholes are considered invalid. If you think a specification is unclear or underspecified, comment on the question instead.
…Try to optimize your score. For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one.
Explanations of your answer make it more interesting to read and are very much encouraged.…Include a short header which indicates the language(s) of your code and its score, as defined by the challenge.
More generally…
…Please make sure to answer the question and provide sufficient detail.
…Avoid asking for help, clarification or responding to other answers (use comments instead).
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f178837%2fincrement-a-guid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
z9KkwyNG0LV7C aPcet,wTZBbvlKlxUTo7i2BZl84Ac sS uN dPIVQg YK5aTRkPT26auzEjHGEcjm,n f0C7KeSSt 81
1
$begingroup$
Are we supposed to leave the 6 fixed bits in the UUIDv4 intact?
$endgroup$
– Filip Haglund
yesterday
2
$begingroup$
@FilipHaglund: No, just treat the GUID as a 128-bit number, where none of the bits have any special meaning. Similarly, GUIDs are assumed to sort in straightforward lexicographical order rather than in the binary order of a Windows
GUID
struct.$endgroup$
– dan04
yesterday
3
$begingroup$
Suggested test case:
89f25f2f-2f7b-4aa6-b9d7-46a98e3cb29f
to ensure that answers can make the transition9 -> a
.$endgroup$
– Kamil Drakari
yesterday
1
$begingroup$
@dana: You may use any data type for which your language's equivalent of C#'s
foreach (char ch in theInput)
is valid.$endgroup$
– dan04
yesterday